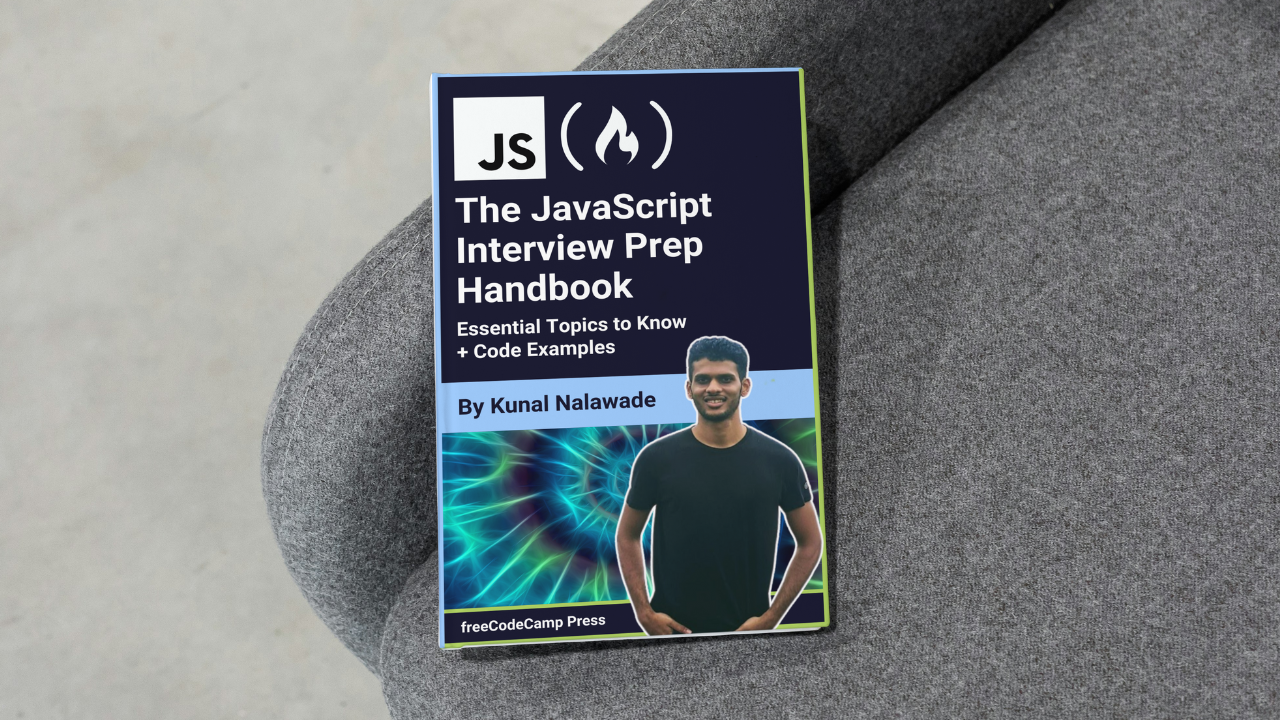
How to Use the async and await Keywords
September 10, 2024About 2 min
How to Use the async and await Keywords κ΄λ ¨
The JavaScript Interview Prep Handbook β Essential Topics to Know + Code Examples
JavaScript is a widely used language in web development and powers interactive features of virtually every website out there. JavaScript makes it possible to create dynamic web pages and is very versatile. JavaScript remains one of the most in-demand programming languages in 2024. Many companies are looking for proficiency in...
The JavaScript Interview Prep Handbook β Essential Topics to Know + Code Examples
JavaScript is a widely used language in web development and powers interactive features of virtually every website out there. JavaScript makes it possible to create dynamic web pages and is very versatile. JavaScript remains one of the most in-demand programming languages in 2024. Many companies are looking for proficiency in...
await
keyword pauses execution of a function till a promise has been resolved or rejected. await
can only be used inside an async
function. Let's take an example:
function dataPromise() {
return new Promise(resolve => {
setTimeout(() => resolve("Data retrieved"), 500);
});
}
async function fetchData() {
try {
const res = await dataPromise();
console.log(res); // Data retrieved (after 500ms)
} catch(err) {
console.log(err);
}
}
fetchData();
When dataPromise()
is called, the execution of the function pauses for 500ms. The execution continues after the promise has resolved. To handle errors, surround the code with a try-catch
block.
The await
keyword also makes it easier to work with multiple promises that run one after the other.
function promise1() {
return new Promise(resolve => {
setTimeout(() => resolve("Promise 1 resolved"), 500);
});
}
function promise2() {
return new Promise(resolve => {
setTimeout(() => resolve("Promise 2 resolved"), 300);
});
}
async function fetchData() {
try {
const res1 = await promise1();
console.log(res1); // Promise 1 resolved (after 500ms)
const res2 = await promise2();
console.log(res2); // Promise 2 resolved (after 300ms)
} catch(err) {
console.log(err);
}
}
fetchData();
async
and await
makes it easier to work with promises and also makes your code cleaner and readable by removing nesting in the code.