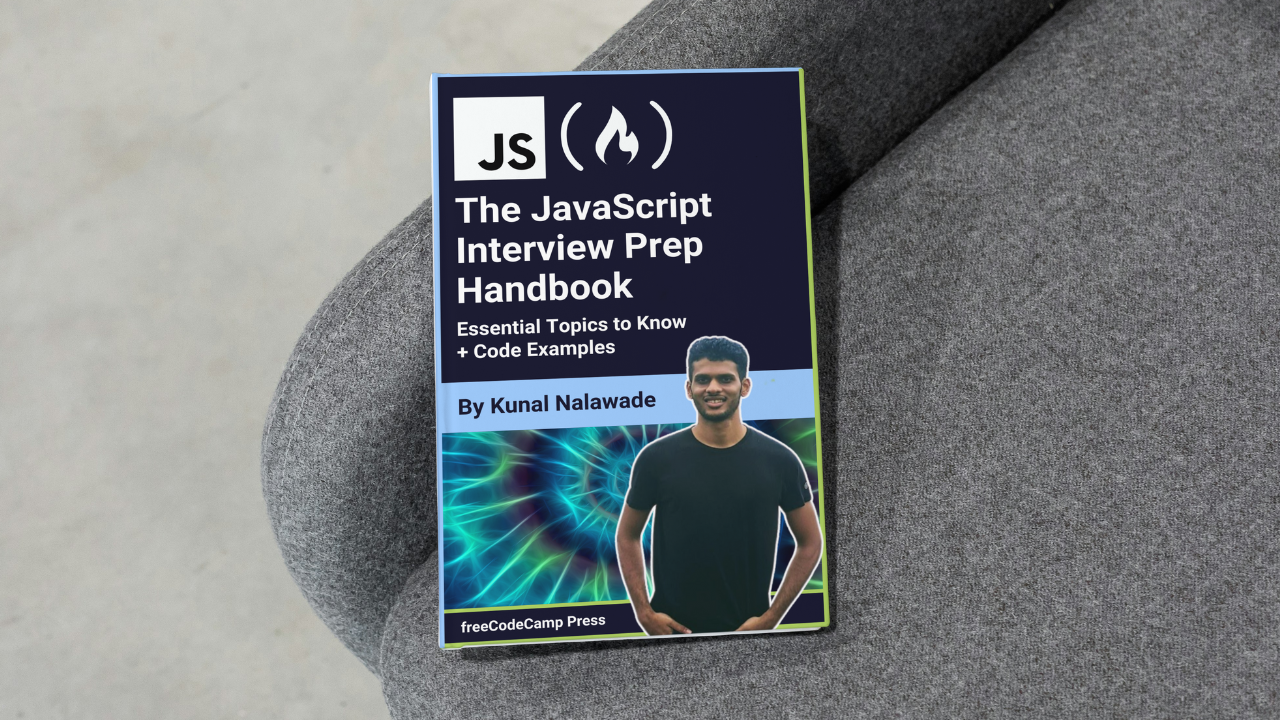
What is Currying?
What is Currying? κ΄λ ¨
Currying is a technique where a function with multiple arguments is transformed into a sequence of functions, with each function taking a single argument and returning another function. For example, consider the function below:
function add(a, b, c) {
return a + b + c;
}
With currying, the above function can be written as:
function curryAdd(a) {
return function(b) {
return function(c) {
return a + b + c;
};
};
}
Here, each function inside curryAdd
takes one argument and returns another function till all arguments are collected. curryAdd
is also known as a higher-order function.
Currying allows you to reuse partial implementations of a function. In case you do not have all the arguments available, you can fix some arguments of the function initially and return a reusable function.
// Reusable function
const addTwo = curryAdd(2);
console.log(addTwo); // prints the function
// Calling final result
const result1 = addTwo(5)(10);
console.log(result1); // 17
const result2 = addTwo(3)(5);
console.log(result2); // 10
addTwo
is a reusable function that can be used later, when additional arguments become available.
Thus, currying enhances code modularity and flexibility with partial function application. It also allows you to create functions that are tailored to specific needs as seen in the example above.
Currying simplifies complex functions by breaking them down into simpler, more manageable parts. This leads to cleaner and readable code.