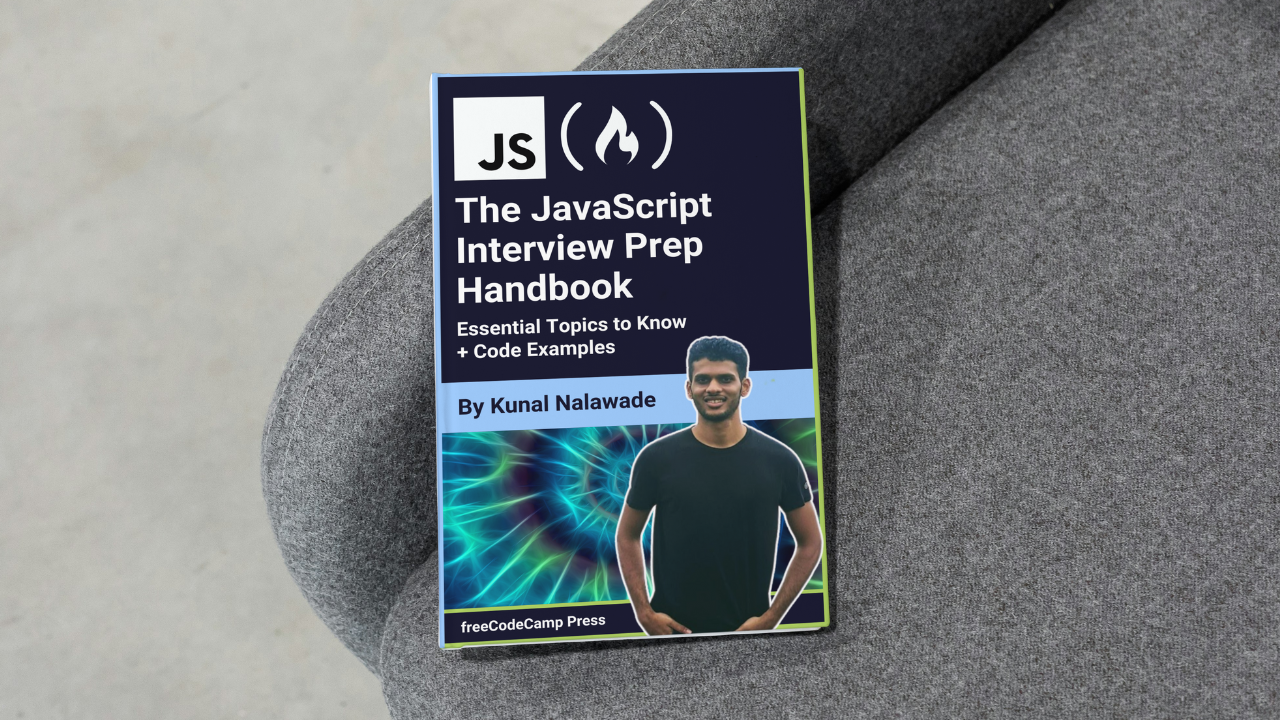
How to Implement Throttling
How to Implement Throttling κ΄λ ¨
Throttling is a technique that limits the rate at which a function is called. A throttled function executes for the first time and can only be called again after a certain delay. If it is called within the delay, nothing happens.
Let's see how to implement it:
function throttle(func, delay) {
let timeout = null;
return (...args) => {
if (!timeout) {
func(...args);
timeout = setTimeout(() => {
timeout = null;
}, delay);
}
};
}
throttle()
takes a function and a delay as parameters, and returns a throttled function. When you call the throttled function, it executes the first time and starts a timeout with delay
. Within this time, no matter how many times you call the throttled function, it won't execute.
Let's test this behavior:
function fun(a, b) {
console.log(`This is a function with arguments ${a} and ${b}`);
}
const throttledFun = throttle(fun, 500);
throttledFun(2, 3); // This is a function with arguments 2 and 3
throttledFun(2, 3);
setTimeout(() => {
throttledFun(2, 3);
}, 300);
setTimeout(() => {
throttledFun(2, 3); // This is a function with arguments 2 and 3
}, 600);
Here, the first call executes immediately, and for the next 500ms, no function call will execute. The last one executes since it is called after 500ms.
Throttling also uses the concept of closures. I have explained throttling in detail in my post, so check it out