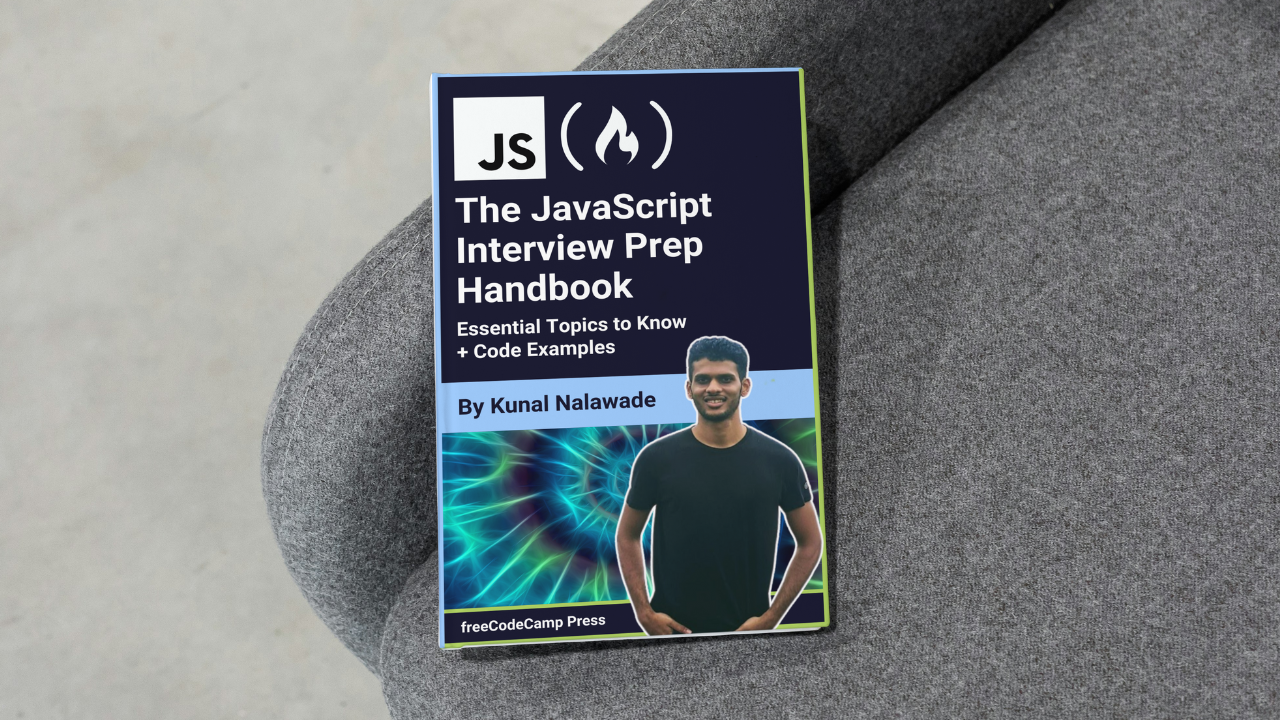
What are Prototypes and Prototypal Inheritance?
What are Prototypes and Prototypal Inheritance? κ΄λ ¨
Inheritance is a concept in object oriented programming that allows an object to inherit properties and methods from another object. However, inheritance works differently in JavaScript.
In JavaScript, every object has a property that links to another object called a prototype. The prototype itself is an object that can have its own prototype, thus forming a prototype chain. This chain ends when we reach a prototype equal to null
.
Prototype allows you to inherit methods and properties from another object. When a property does not exist on an object, JavaScript searches its prototype and so on till it reaches the end of the prototype chain.
Let's understand with an example.
let animal = {
eats: true,
walk() {
console.log("Animal is walking");
}
};
const rabbit = Object.create(animal);
rabbit.jumps = true;
rabbit.walk(); // Animal is walking
Object.create
creates a new object rabbit
with its prototype set to animal
. You can also set additional properties of the new object.
Also, the walk()
method does not exist on rabbit
, so it searches the object's prototype animal
. This means the rabbit
object has inherited the properties and methods of the animal
object.
You can also use the ES6 Object.setPrototypeOf
method on any object.
const dog = {
bark() {
console.log("Dog barking");
}
};
Object.setPrototypeOf(dog, animal);
console.log(dog.eats); // true
dog.walk(); // Animal is walking
You can also use a function as a constructor and set its prototype using the prototype
property.
function Animal(name) {
this.name = name;
}
Animal.prototype.walk = function () {
console.log(`${this.name} is walking`);
};
const dog = new Animal("Dog");
console.log(dog); // Animal { name: 'Dog' }
dog.walk(); // Dog is walking
You can learn more about prototypes and inheritance in JavaScript in the following post by GermΓ‘n Cocca (gercocca
).