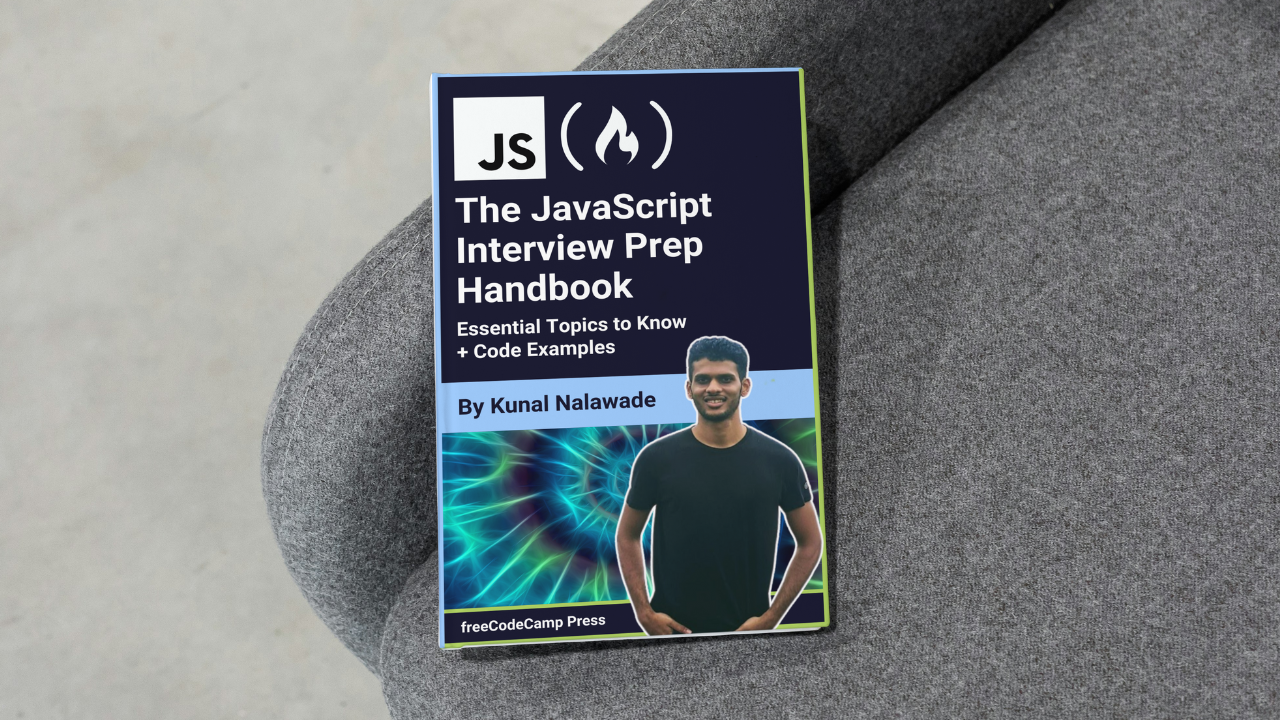
How to Implement Debouncing
How to Implement Debouncing κ΄λ ¨
Debouncing is a technique that delays a function call by few seconds and ensures that there is always a delay between function call and execution.
When you call a function, it gets executed after a delay. However, if you call the function again within that delay, the previous call is cancelled and a new timer is started. The same process repeats for each subsequent function call.
Let's see how to implement it:
function debounce(func, delay) {
let timeout = null;
return (...args) => {
if (timeout) clearTimeout(timeout);
timeout = setTimeout(() => {
func(...args);
timeout = null;
}, delay);
};
}
Debouncing takes a function and a delay as parameters, and returns a new, debounced function. When you call the debounced function, it will execute after delay
milliseconds. If the function is called within that time, it cancels the previous call and waits for delay
again.
Let's test this behavior:
function fun(a, b) {
console.log(`This is a function with arguments ${a} and ${b}`);
}
const debouncedFun = debounce(fun, 500);
debouncedFun(2, 3);
debouncedFun(2, 3);
debouncedFun(2, 3); // This is a function with arguments 2 and 3
The first two calls do not execute, while the third one does, after 500ms. Debouncing uses the concept of closures, so it's important to understand them first.
Debouncing has plenty of applications, with the most popular one being the auto-complete functionality in search bars. I have explained debouncing in detail in the following post