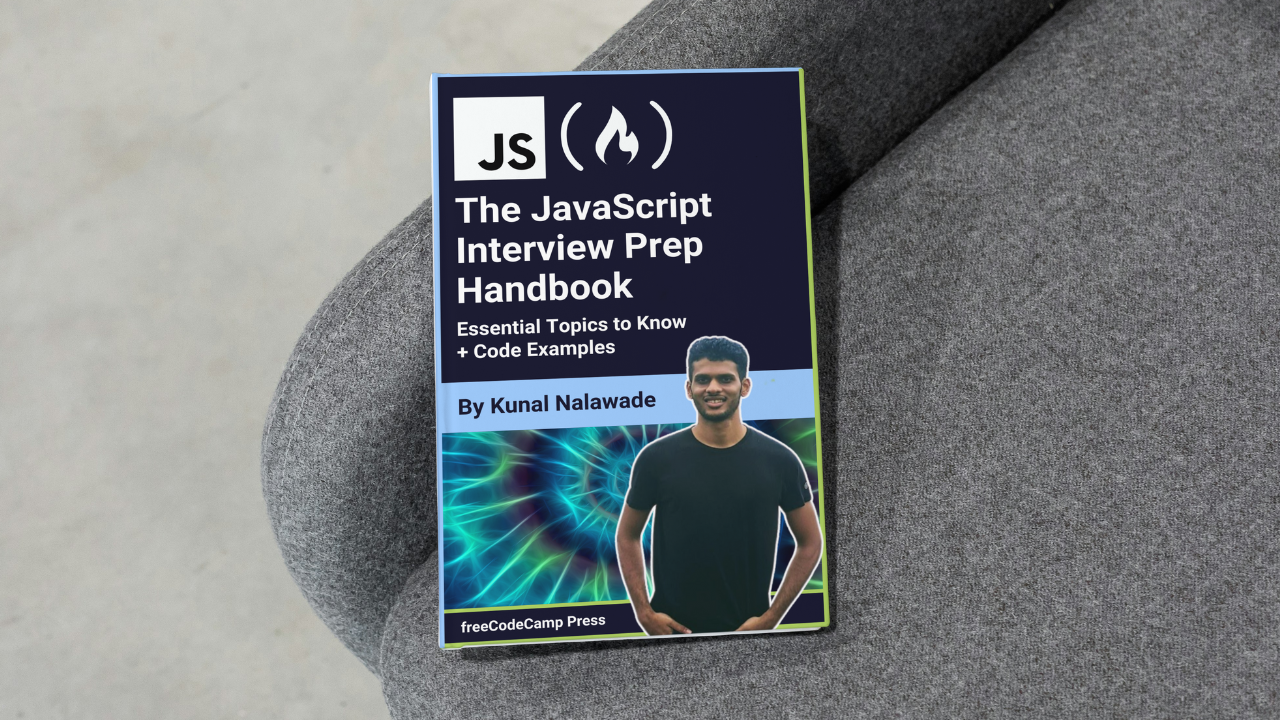
How to Use var, let, and const Keywords.
How to Use var, let, and const Keywords. κ΄λ ¨
In JavaScript, you can declare a variable in three ways: using var
, let
and const
. It's essential to understand the difference between these three.
A var
variable has global and function level scope. If the variable is declared globally, it can be accessed anywhere and if declared inside a function, it can be accessed anywhere within the function.
var a=5
function fun() {
var b=4
}
console.log(a) // 5
console.log(b) // throws ReferenceError
A let
variable has block level scope. This variable, if declared inside a block, cannot be accessed outside it. For example:
var a = 5;
if (a > 1) {
var b = 6;
let c = 7;
}
console.log(a); // prints 5
console.log(b); // prints 6
console.log(c); // throws ReferenceError
Here, variables a
and b
have global scope, so they can be accessed anywhere. Variable c
cannot be accessed outside the if
block since let
only has block level scope.
const
is used to declare a constant. Once a variable is declared with const
, it cannot be modified.
const x = 5;
x = 6; // Throws an error
However, you can modify properties of an object, or elements of an array.
const obj = { name: 'kunal', age: 21 };
obj.name = 'alex';
console.log(obj); // { name: 'alex', age: 21 }
const arr = [1, 2, 3];
arr[1] = 4;
console.log(arr); // [ 1, 4, 3 ]