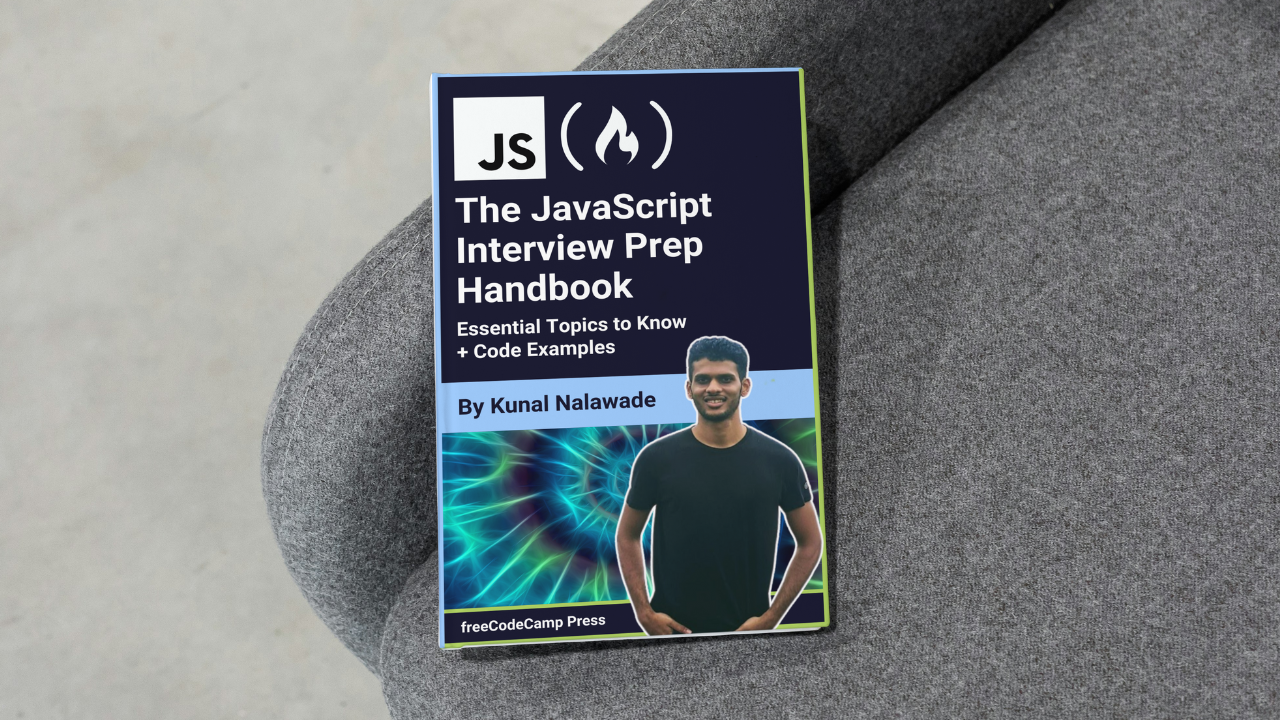
How to Implement Polyfills for Array.map(), Array.reduce(), and Array.filter()
How to Implement Polyfills for Array.map(), Array.reduce(), and Array.filter() κ΄λ ¨
JavaScript has evolved a lot since its inception. Several methods and constructs have been added to JavaScript that didn't exist before. Most modern browsers use the latest versions of JavaScript.
However, there are several applications still running on older browsers that use older versions of JavaScript . Array methods like map
, reduce
and filter
may not be available. Therefore, you may have to provide polyfills for these methods.
Polyfills are pieces of code that provide modern functionality to older browsers that don't support it. This ensures that your code runs seemlessly on different browsers and versions.
Most companies have websites that still cater to users and systems running old browsers. So, knowing how to write polyfills for frequently used methods is important.
In our case, we are going to write polyfills for Array.map
, Array.reduce
and Array.filter
methods. This means we are going to write our own implementations instead of using the default ones.
Array.map
This method takes a callback function as a parameter, executes it on each array element and returns a new, modified array.
The callback function takes three arguments: the array element, index and the array itself. The last two arguments are optional.
Array.prototype.map = function(callback) {
var newArray = [];
for (var i = 0; i < this.length; i++) {
newArray.push(callback(this[i], i, this));
}
return newArray;
};
βThe logic is simple. Call the function for each element of the array and append each value to the new array. The this
keyword is the object on which you are calling the function, in this case, the array.
Array.filter
This method also takes a callback function as a parameter. The callback function runs a condition on each array element and returns a Boolean value. The filter
method returns a new, filtered array containing elements that satisfy the condition.
This callback function takes three arguments: the array element, index and the array itself. The last two arguments are optional.
Array.prototype.filter = function(callback) {
var filteredArr = [];
for (var i = 0; i < this.length; i++) {
var condition = callback(this[i], i, this);
if (condition) {
filteredArr.push(this[i]);
}
}
return filteredArr;
};
Here, use the Boolean value returned by the callback function to add elements to the new array.
Array.reduce
This method takes a callback function and an initial value as parameters and reduces the array to a single value. This is done by executing the function on the accumulator and current value and storing the result into the accumulator.
The callback function takes four arguments: the accumulator, current element, index and array itself. The last two arguments are optional.
Array.prototype.reduce = function(callback, initialValue) {
var accumulator = initialValue;
for (var i = 0; i < this.length; i++) {
if (accumulator !== undefined) {
accumulator = callback(accumulator, this[i], i, this);
} else {
accumulator = this[i];
}
}
return accumulator;
};
Initially, set the accumulator to the initial value. Execute the callback function for each array element and store the result in accumulator. If the accumulator is undefined, then set it to the element itself.
Let's test these methods:
const arr = [1, 2, 3];
console.log(arr.map(ele => ele * 2)); // [ 2, 4, 6 ]
console.log(arr.filter(ele => ele < 2)); // [ 1 ]
console.log(arr.reduce((total, ele) => total + ele, 0)); // 6
Note
Before adding a polyfill for any property, always check if the property exists on the object's prototype, or you might override the existing behaviour. For example:
if (!Array.prototype.map)