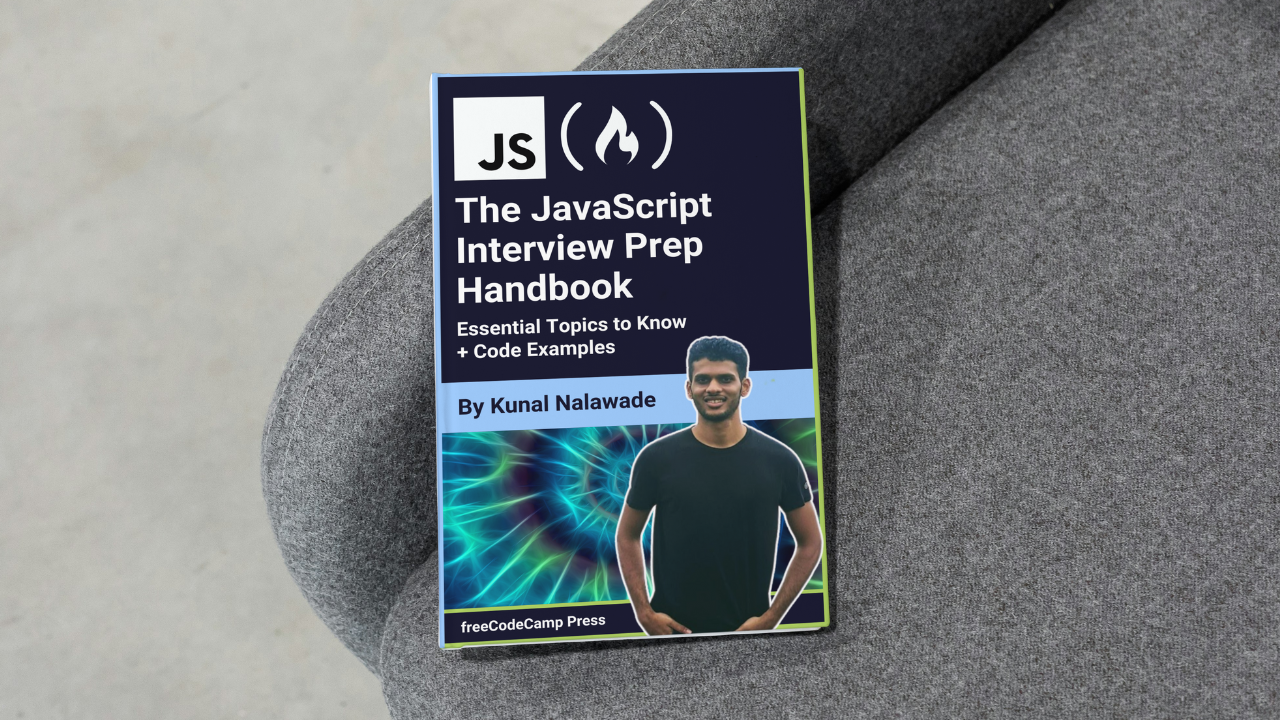
How to Use the call, apply and bind Methods.
How to Use the call, apply and bind Methods. 관련
When you use this
inside a function, its value is set to the object on which the function is called. Let's take an example:
function getInfo() {
console.log(`Name: ${this.name}, Age: ${this.age}`);
}
call
, apply
and bind
are used to set the value of the this
keyword inside a method.
call
To call getInfo()
function on an object, use the call
function. Let's create two objects and call getInfo()
on these objects.
const ob1 = { name: 'alex', age: 25 };
const ob2 = { name: 'marcus', age: 23 };
getInfo.call(ob1); // Name: alex, Age: 25
getInfo.call(ob2); // Name: marcus, Age: 23
call
sets the value of the this
keyword inside a function.
apply
The apply
method is similar to call
, but it differs in the way you pass arguments. Consider a function with arguments:
function getInfo(a, b) {
console.log(`Name: ${this.name}, Age: ${this.age}`);
console.log(`Args: ${a} and ${b}`);
}
const obj = {
name: 'alex',
age: 25
};
getInfo.call(obj, 2, 3);
getInfo.apply(obj, [2, 3]);
bind
bind
is used to create a new function that has its this
keyword set to one object. Let's use the above getInfo
function as example.
const obj = {
name: 'alex',
age: 25
};
const objGetInfo = getInfo.bind(obj, 2, 3);
objGetInfo();
When bind
is called on getInfo()
function, it returns a new function that is bound to obj
. Now, every time you call the objGetInfo()
function, this
keyword refers to obj
.
All three methods are similar. That is, they set the value of this
keyword. However, a key difference in bind
is that it returns a new function, whereas call
and apply
simply just call the function.