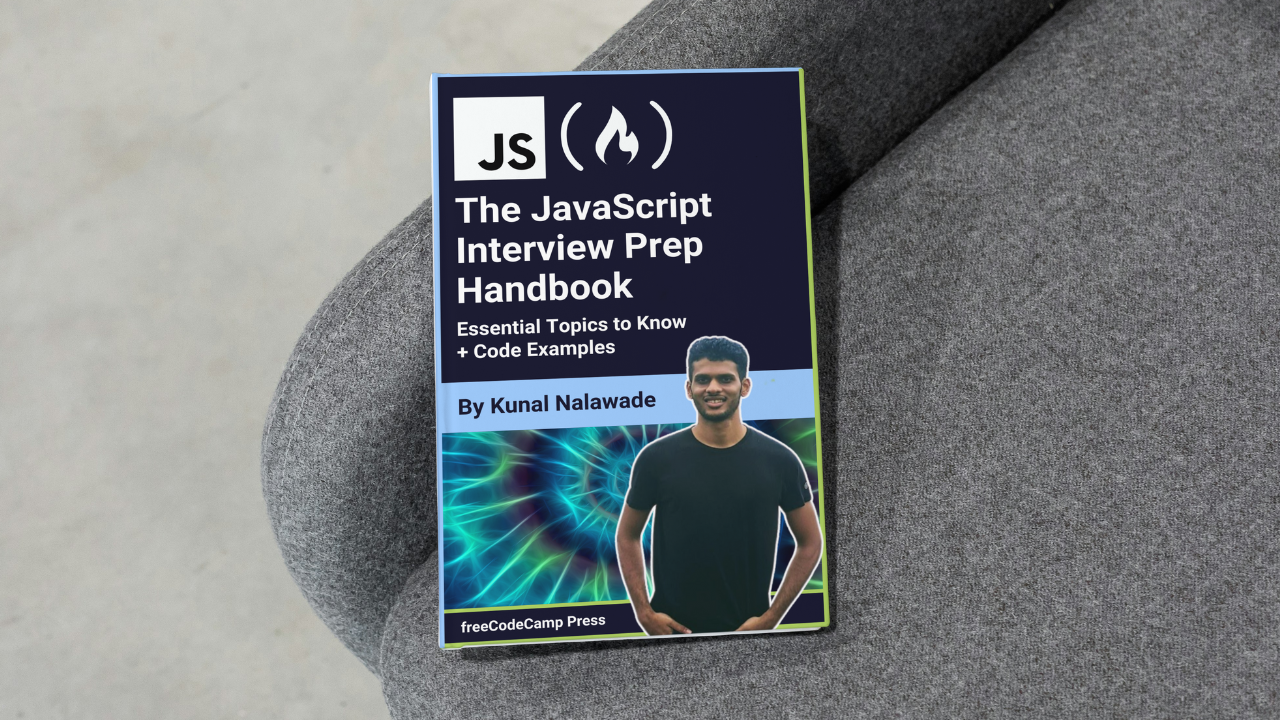
How Event Propagation Works β Bubbling and Capturing.
How Event Propagation Works β Bubbling and Capturing. κ΄λ ¨
Event propagation takes place when an event is captured and handled by the target element and all its ancestors. Take the following example:
<body>
<div id="box"> <button id="button">Click Me</button> </div>
<script src="script.js"></script>
</body>
When you click the button, you have also clicked the div
element as well the body
. The event is propagated throughout the DOM tree. Let's add handlers to all the above elements:
document.body.addEventListener("click", () => {
console.log("Body clicked");
});
document.getElementById("box").addEventListener("click", () => {
console.log("div clicked");
});
document.getElementById("button").addEventListener("click", () => {
console.log("Button clicked");
});
Event propagation occurs in two ways:
Event Bubbling
When the button is clicked, the event handler of the button is called first. Then, the event bubbles up the DOM tree and the event handlers of parents are called sequentially from the immediate parent to the highest ancestor. That is: the div
and body
elements respectively.
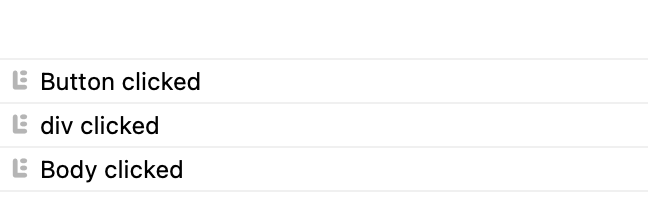
βEvent Capturing
This works similar to event bubbling, but in reverse. The event is first captured by the root element, then travels down the DOM tree to the target element.
The event handlers are called in sequence starting from the root element, down to the target element. This can be achieved by passing true
as the third parameter in the addEventListener()
function.β
document.body.addEventListener("click", () => {
console.log("Body clicked");
}, true);
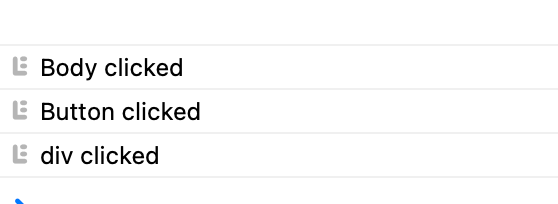
However, this looks counter-productive. After all, the user only wants to click the button, they have no idea of the DOM tree structure. So, to prevent this behaviour, we can use the stopPropagation()
method.
document.getElementById("button").addEventListener("click", (event) => {
event.stopPropagation();
console.log("Button clicked");
});
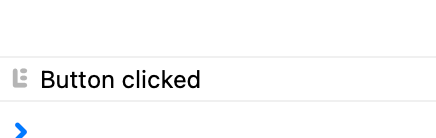