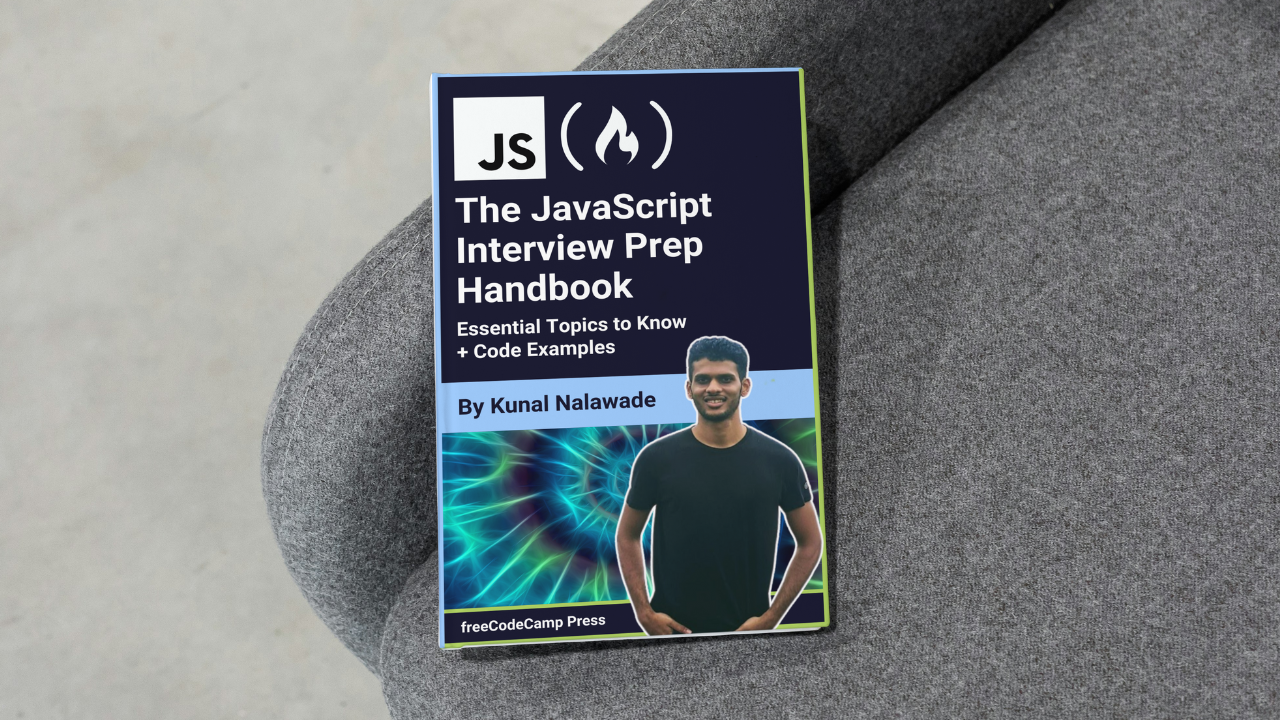
βHow to Use the Spread Operator
September 10, 2024About 1 min
βHow to Use the Spread Operator κ΄λ ¨
The JavaScript Interview Prep Handbook β Essential Topics to Know + Code Examples
JavaScript is a widely used language in web development and powers interactive features of virtually every website out there. JavaScript makes it possible to create dynamic web pages and is very versatile. JavaScript remains one of the most in-demand programming languages in 2024. Many companies are looking for proficiency in...
The JavaScript Interview Prep Handbook β Essential Topics to Know + Code Examples
JavaScript is a widely used language in web development and powers interactive features of virtually every website out there. JavaScript makes it possible to create dynamic web pages and is very versatile. JavaScript remains one of the most in-demand programming languages in 2024. Many companies are looking for proficiency in...
The spread operator is used to spread out contents of an array or object into individual elements or collect a bunch of elements into a single object. It has following use cases:
Spread operator can be used to copy an array into a new one:
const arr1 = [2, 4, 5];
const arr2 = [...arr1];
console.log(arr1); // [2, 4, 5]
console.log(arr2); // [2, 4, 5]
console.log(arr1 == arr2); // false
arr1
and arr2
are completely different objects as shown with the equality operator.
You can also reuse fields from one object while creating a new object:
const obj1 = { name: 'kunal', age: 23 };
const obj2 = { ...obj1, gender: 'male', city: 'Mumbai' };
console.log(obj2); // { name: 'kunal', age: 23, gender: 'male', city: 'Mumbai' }
You can collect multiple arguments passed to a function into an array.
function fun1(...args) {
console.log(args);
}
fun1(1, 2, 3, 4, 5); // [ 1, 2, 3, 4, 5 ]
Or you can pass elements of an array as individual arguments to a function.
function fun2(a, b) {
console.log(`${a} and ${b}`);
}
const numbers = [1, 2];
fun2(...numbers);