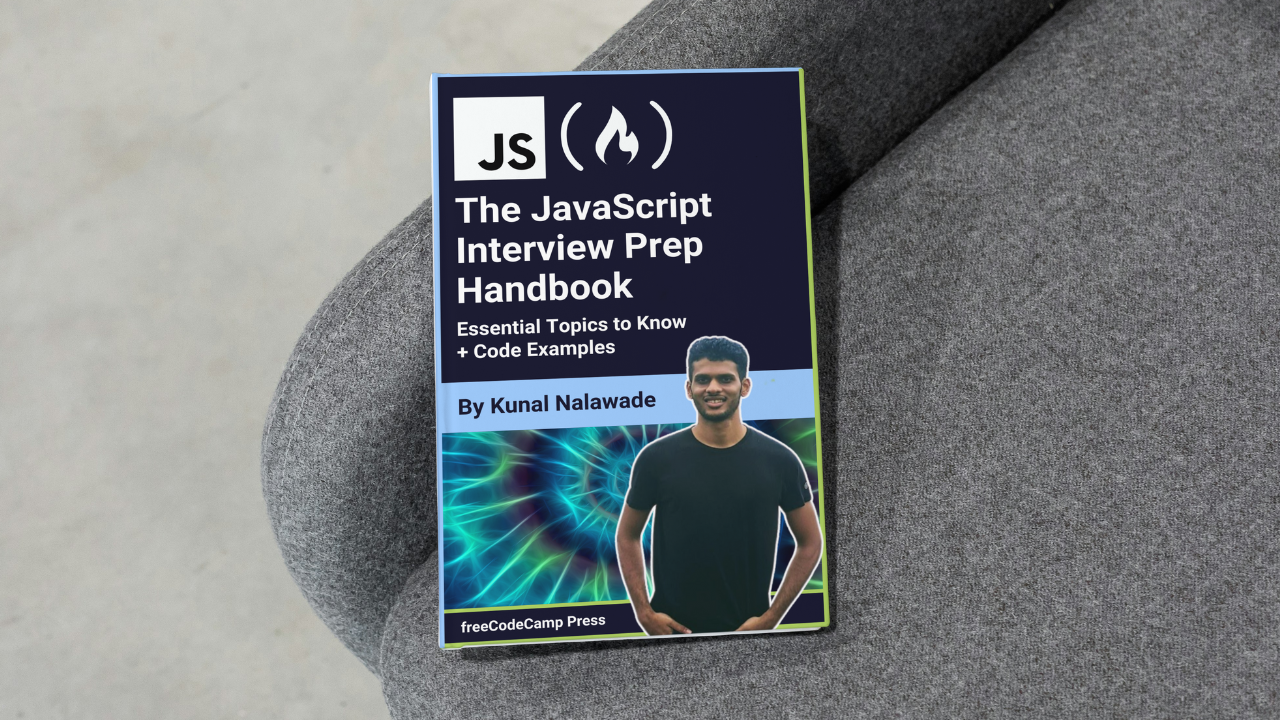
What are Generator Functions?
What are Generator Functions? 관련
Generator functions are special type of functions that can pause their execution and resume it later. They also return a value each time they pause execution.
Generator functions can be used to return a sequence of values in an iterative manner as opposed to normal functions that return only once.
Following is a basic example of a generator function:
function* generatorFunction() {
yield 1;
yield 2;
}
A generator function is declared with function*
and the yield
keyword is used to pause execution and return a value. The above syntax creates a GeneratorFunction object.
const gen = generatorFunction()
This object uses an iterator to execute a generator function. The iterator provides a next()
method that executes the function's body till the next yield statement and returns an object containing the yielded value and a done
property (Boolean), which indicates if the generator function has reached its end.
Let's call the generator function:
console.log(gen.next().value); // 1
console.log(gen.next()); // { value: 2, done: false }
console.log(gen.next()); // { value: undefined, done: true }
Here, the first call to next()
yields 1 and the second one yields 2. The last one yields nothing and sets the done
flag to true as there are no more yield
statements.
You can also loop over a generator function using for
loop:
for(value of generatorFunction()) {
console.log(value)
}
In this way, you can control the execution of a generator function by entering and exiting a function at any time.