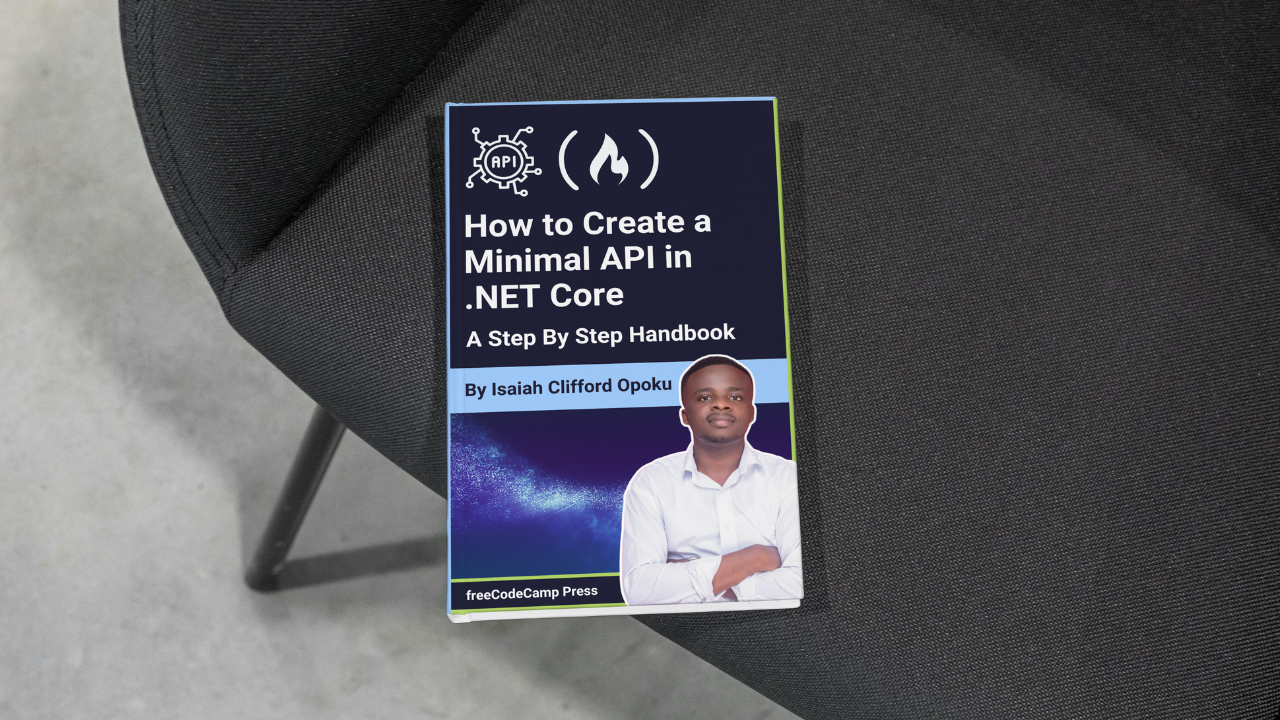
How to Create a Contract
How to Create a Contract κ΄λ ¨
Contracts are Data Transfer Objects (DTOs) that define the structure of the data exchanged between the client and the server. In our application, we will create contracts to represent the data sent and received by our API endpoints.
Here are the contracts we are going to create:
- CreateBookRequest: This represents the data sent when creating a new book.
- UpdateBookRequest: tHI Represents the data sent when updating an existing book.
- BookResponse: Represents the data returned when retrieving a book.
- ErrorResponse: Represents the error response returned when an exception occurs.
- ApiResponse: Represents the response returned by the API.
In the Contracts
folder, create a new file named CreateBookRequest
and add the following code:
namespace bookapi_minimal.Contracts
{
public record CreateBookRequest
{
public string Title { get; init; }
public string Author { get; init; }
public string Description { get; init; }
public string Category { get; init; }
public string Language { get; init; }
public int TotalPages { get; init; }
}
}
In the Contracts
folder, create a new file named UpdateBookRequest
and add the following code:
namespace bookapi_minimal.Contracts
{
public record UpdateBookRequest
{
public string Title { get; set; }
public string Author { get; set; }
public string Description { get; set; }
public string Category { get; set; }
public string Language { get; set; }
public int TotalPages { get; set; }
}
}
In the Contracts
folder, create a new file named BookResponse
and add the following code:
namespace bookapi_minimal.Contracts
{
public record BookResponse
{
public Guid Id { get; set; }
public string Title { get; set; }
public string Author { get; set; }
public string Description { get; set; }
public string Category { get; set; }
public string Language { get; set; }
public int TotalPages { get; set; }
}
}
In the Contracts
folder, create a new file named ErrorResponse
and add the following code:
// Contracts/ErrorResponse.cs
namespace bookapi_minimal.Contracts
{
public record ErrorResponse
{
public string Title { get; set; }
public int StatusCode { get; set; }
public string Message { get; set; }
}
}
In the Contracts
folder, create a new file named ApiResponse
and add the following code:
namespace bookapi_minimal.Contracts
{
public class ApiResponse<T>
{
public T Data { get; set; }
public string Message { get; set; }
public ApiResponse(T data, string message)
{
Data = data;
Message = message;
}
}
}
These contracts help us define the structure of the data exchanged between the client and the server, making it easier to work with the data in our application.
In the next section, we will create services to implement the business logic of our application.