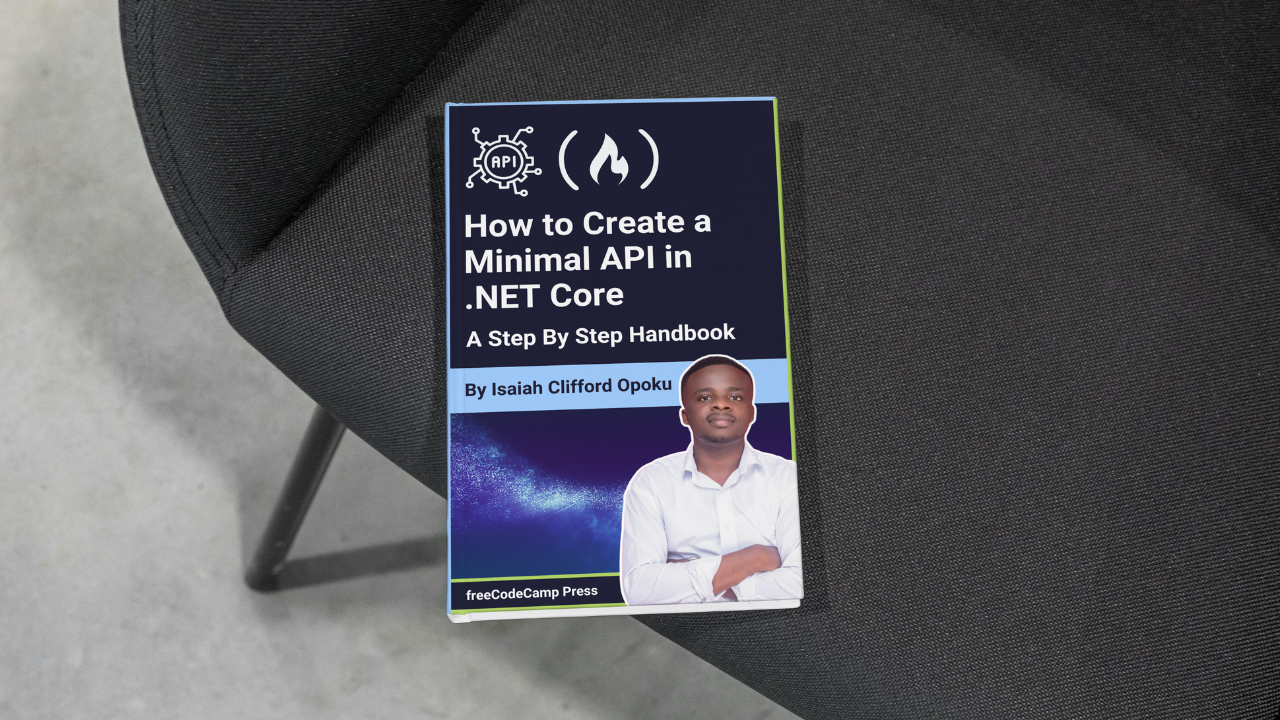
How to Test the API Endpoints
How to Test the API Endpoints κ΄λ ¨
Now we can test our endpoints using Swagger. To do this, run the application by executing the following command in the terminal:
dotnet run
This will run our application. You can open your browser and navigate to https://localhost:5001/swagger/index.html
to access the Swagger documentation. You should see a list of API endpoints, request and response models, and the ability to test the endpoints directly from the browser.
If your port number is different from 5001
, don't worry β it will still work. The port might change depending on the type of machine you're using, but it will still achieve the same result.
How to Test the Get All Books
Endpoint
To test the Get All Books
endpoint, follow these steps:
- In the Swagger documentation, click on the
GET /api/v1/books
endpoint. - Click the
Try it out
button. - Click the
Execute
button.
This will send a request to the API to retrieve all the books in the database.
You should see the response from the API, which will include the list of books that were seeded in the database.
The image below shows the response from the API:
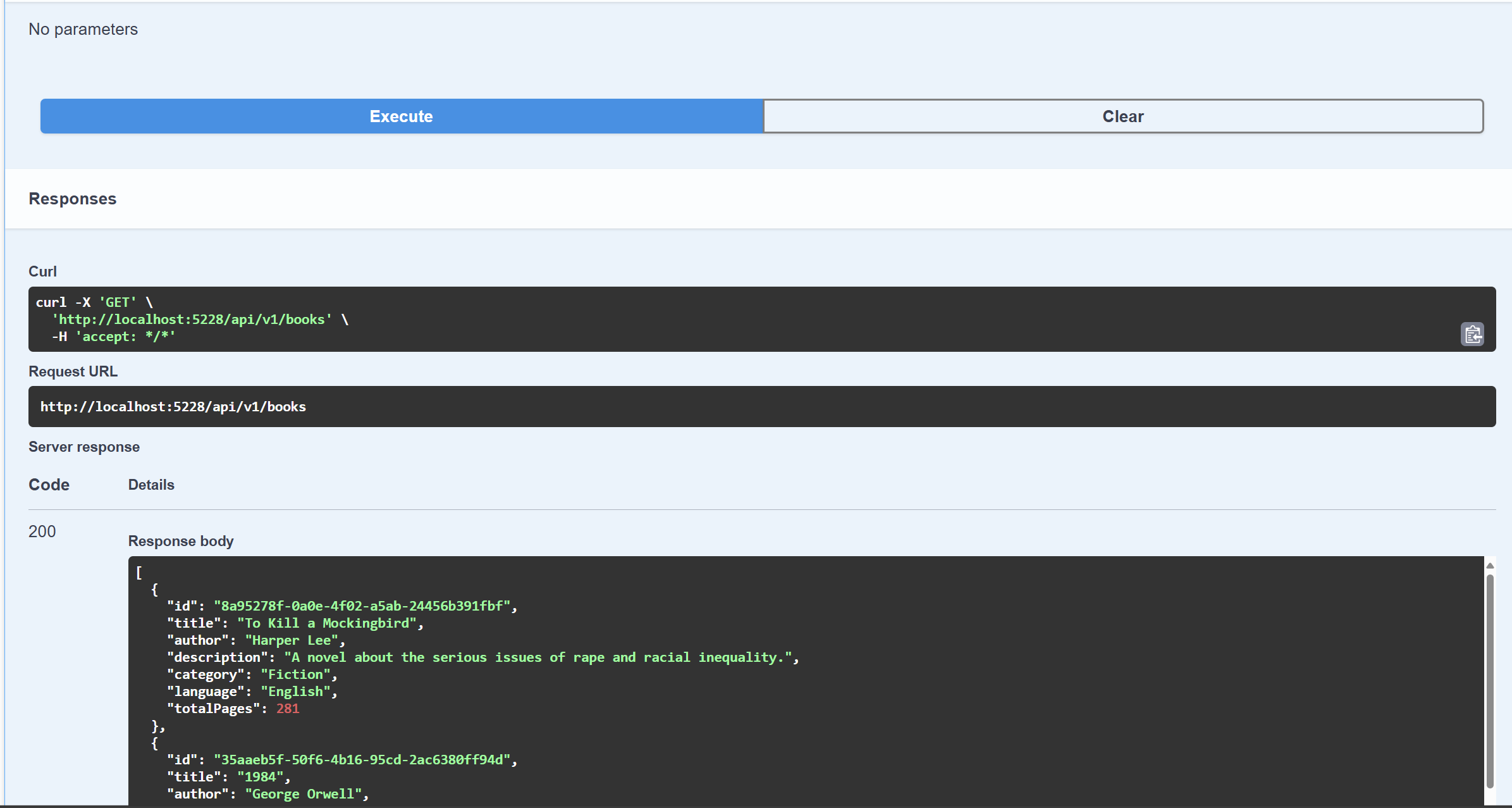
How to Test the Get Book by ID
Endpoint
To test the Get Book by ID
endpoint, follow these steps:
- In the Swagger documentation, click on the
GET /api/v1/books/{id}
endpoint. - Enter the ID of a book in the
id
field. You can use one of the book IDs that was seeded in the database. - Click the
Try it out
button.
This will send a request to the API to retrieve the book with the specified ID. You should see the response from the API, which will include the book with the specified ID.
The image below shows the response from the API:

How to Test the Add Book
Endpoint
To test the Add Book
endpoint, follow these steps:
- In the Swagger documentation, click on the
POST /api/v1/books
endpoint. - Click the
Try it out
button. - Enter the book details in the request body.
- Click the
Execute
button.
This will send a request to the API to add a new book to the database.
You should see the response from the API, which will include the newly created book.
The image below shows the response from the API:
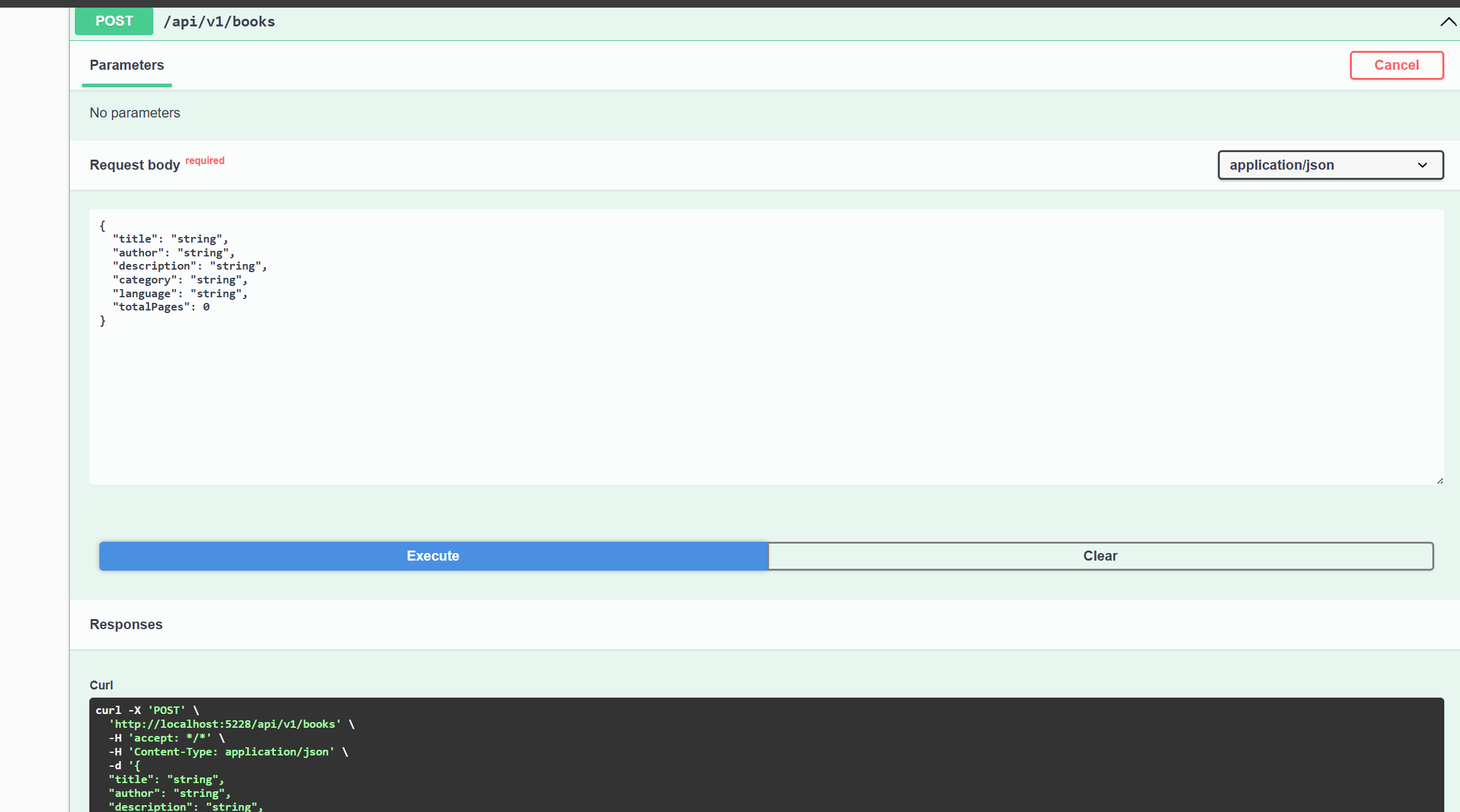
How to Test the Update Book
Endpoint
To test the Update Book
endpoint, follow these steps:
- In the Swagger documentation, click on the
PUT /api/v1/books/{id}
endpoint. - Enter the ID of a book in the
id
field. You can use the id of one of the books that we just added. - Click the
Try it out
button.
This will send a request to the API to update the book with the specified ID.
You should see the response from the API, which will include the updated book.
The image below shows the response from the API:
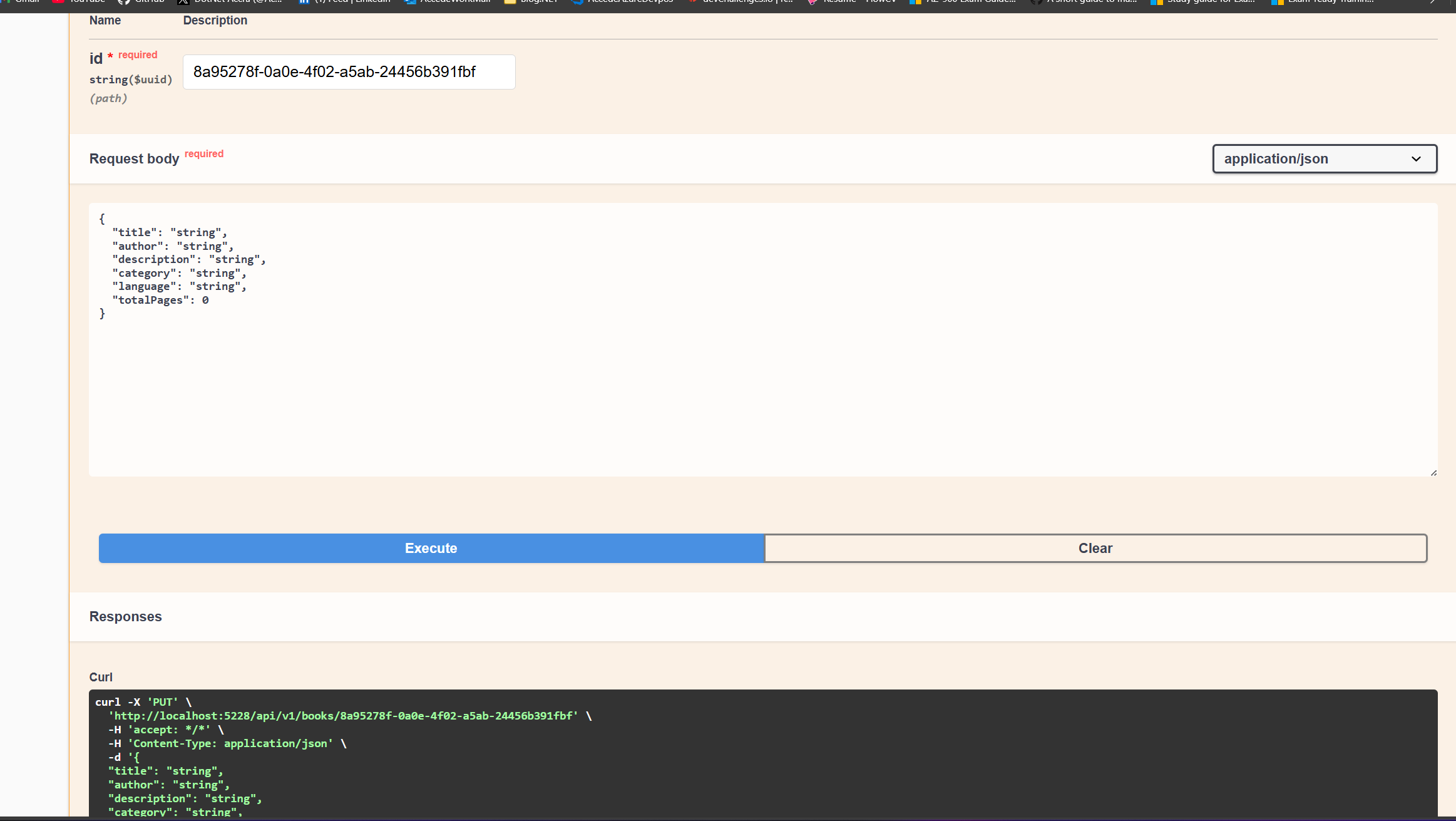
How to Test the Delete Book
Endpoint
To test the Delete Book
endpoint, follow these steps:
- In the Swagger documentation, click on the
DELETE /api/v1/books/{id}
endpoint. - Enter the ID of a book in the
id
field. You can use any of the ids from the books that we just added or the seeded data. - Click the
Try it out
button.
This will send a request to the API to delete the book with the specified ID.
The image below shows the response from the API:
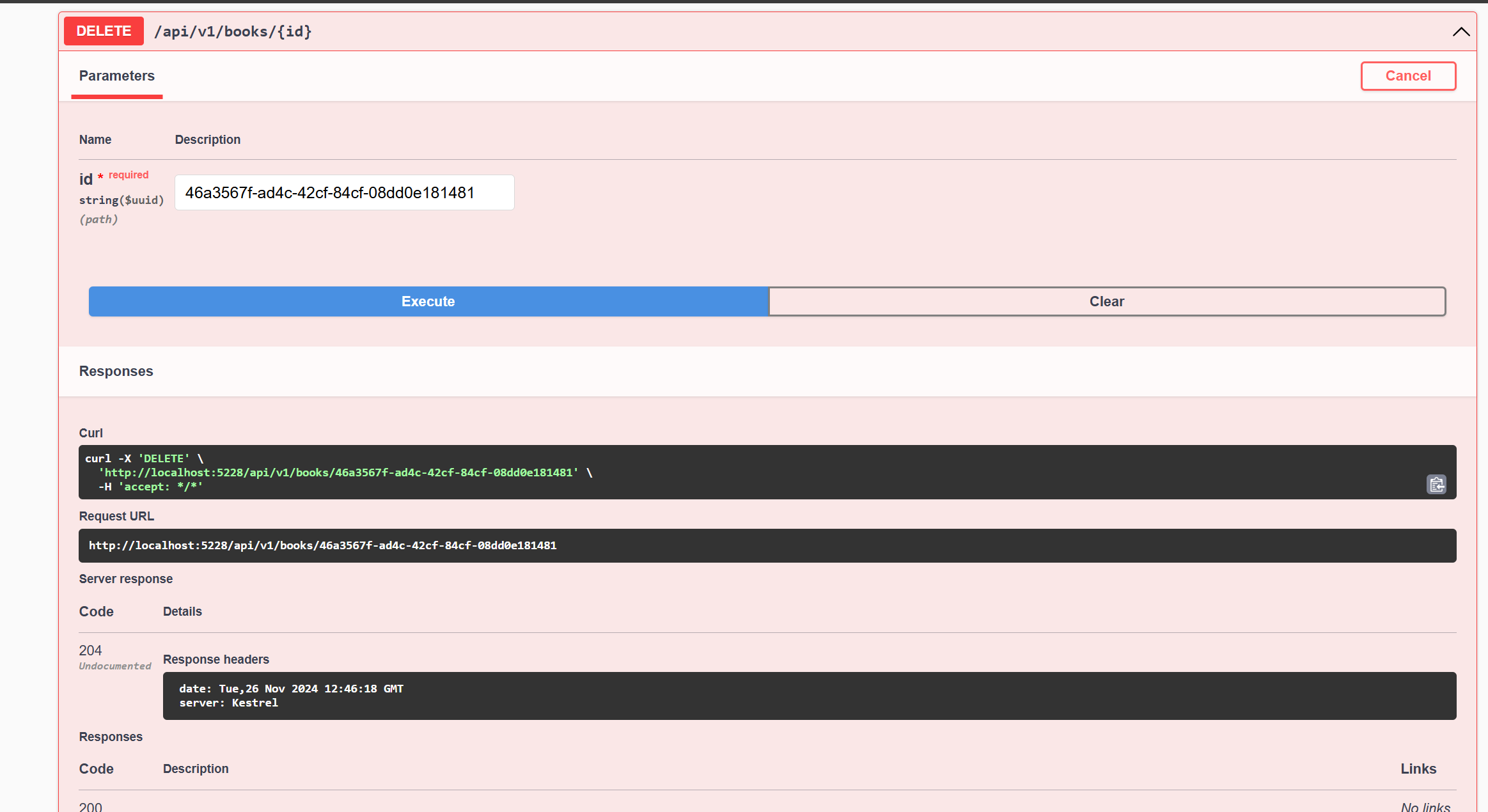
Congratulations! You have implemented all the CRUD operations for books and tested the API endpoints using Swagger, verifying that they work as expected. You can now build on this foundation to add more features and functionality to your API.