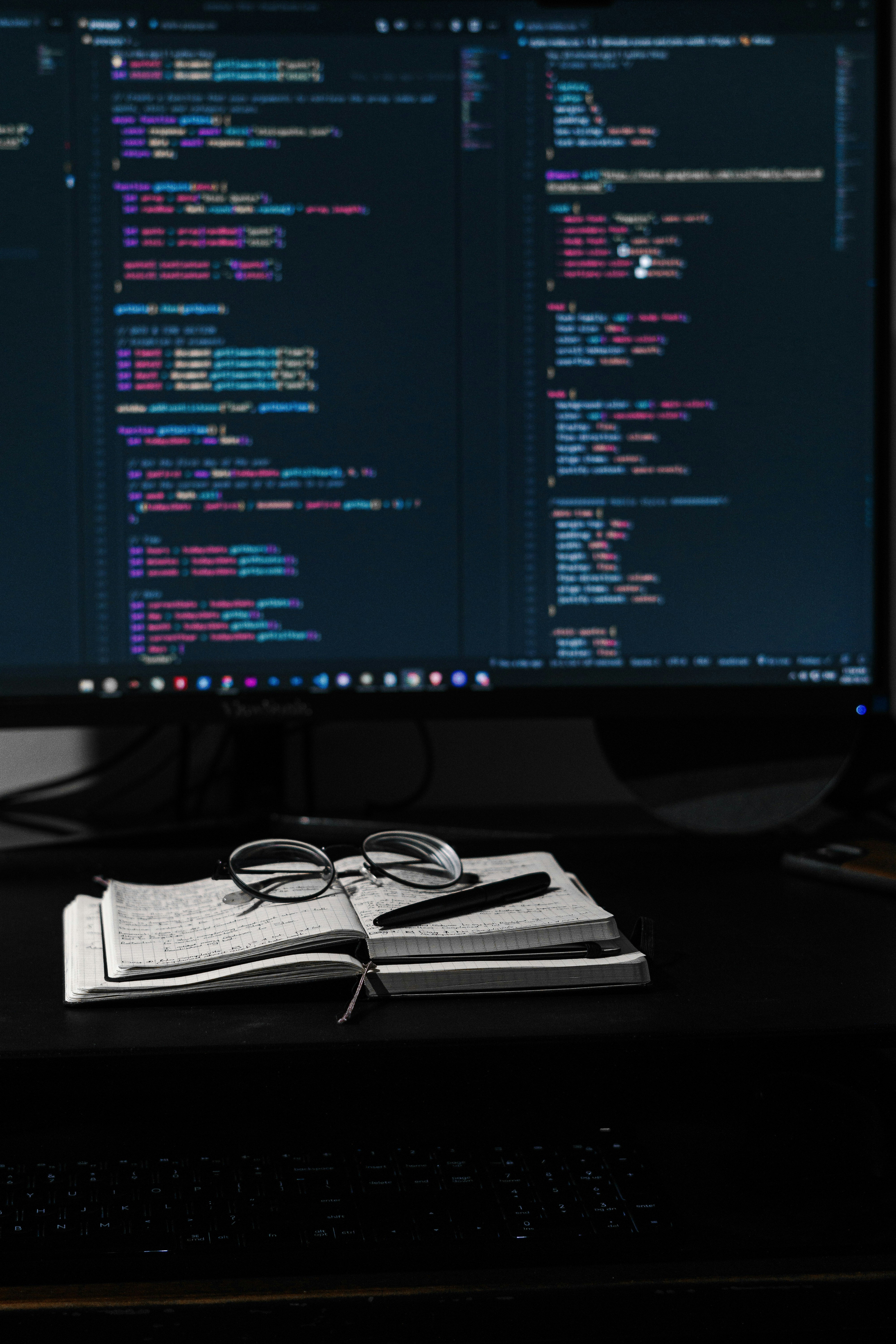
10. Testing and Frameworks
10. Testing and Frameworks ๊ด๋ จ
Testing is an integral part of software development, ensuring that applications behave as expected and reducing the likelihood of bugs. Both Python and JavaScript have robust ecosystems for testing, offering various frameworks and tools to streamline the process.
Popular Testing Frameworks: Mocha/Chai vs. Pytest/Unittest
Both Python and JavaScript have multiple testing frameworks, each tailored to specific needs. For JavaScript, Mocha and Chai are popular choices, while Python developers often use Pytest or the built-in Unittest module.
JavaScript: Mocha and Chai
Mocha is a flexible testing framework for JavaScript, and Chai is often paired with it to provide assertion libraries for more readable test cases.
Example: Mocha and Chai
const { expect } = require('chai');
// Function to test
function add(a, b) {
return a + b;
}
// Mocha test
describe('Add Function', () => {
it('should return the sum of two numbers', () => {
expect(add(2, 3)).to.equal(5);
});
it('should handle negative numbers', () => {
expect(add(-2, -3)).to.equal(-5);
});
});
Python: Pytest
Pytest is a widely used framework in Python that emphasizes simplicity and flexibility. Tests can be written as plain functions, and Pytestโs built-in fixtures streamline setup and teardown.
Example: Pytest
import pytest
# Function to test
def add(a, b):
return a + b
# Pytest functions
def test_add_positive_numbers():
assert add(2, 3) == 5
def test_add_negative_numbers():
assert add(-2, -3) == -5
Key Differences:
- Syntax: Mocha/Chai uses JavaScript syntax with chaining assertions (
expect
), while Pytest relies on Pythonโsassert
keyword. - Fixtures: Pytest fixtures simplify test setup, whereas Mocha relies on manual setup functions (
before
,beforeEach
).
Writing Unit Tests and Test Coverage
Unit testing focuses on verifying individual components or functions in isolation. Both Python and JavaScript frameworks support unit tests, but the tools for measuring test coverage differ.
JavaScript: nyc (Istanbul)
The nyc
tool, built on Istanbul, is commonly used to measure test coverage in JavaScript projects.
Example: Generating Coverage Reports with Mocha and nyc
npm install --save-dev mocha nyc
Add a test script to package.json
:
"scripts": {
"test": "mocha",
"coverage": "nyc mocha"
}
Run the coverage command:
npm run coverage
This generates a report showing which parts of the code were covered during tests.
Python: Coverage.py
In Python, coverage.py
is the standard tool for measuring test coverage.
Example: Generating Coverage Reports with Pytest and Coverage.py
pip install pytest coverage
Run tests with coverage:
coverage run -m pytest
coverage report
This displays coverage percentages for each file and highlights untested lines.
Key Differences:
- JavaScript tools like nyc integrate easily with CI/CD pipelines, while
coverage.py
provides detailed line-by-line reports.
Automation and CI/CD Compatibility
Modern development workflows often include automated testing integrated into CI/CD pipelines. Both Python and JavaScript testing frameworks are compatible with CI/CD tools like Jenkins, GitHub Actions, and GitLab CI.
Example: Automating Tests in a CI/CD Pipeline
JavaScript (GitHub Actions):
name: Node.js CI
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm install
- run: npm test
- run: npm run coverage
Python (GitHub Actions):
name: Python CI
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-python@v2
with:
python-version: '3.9'
- run: pip install -r requirements.txt
- run: pytest --cov=.
Integration and End-to-End Testing
In addition to unit testing, both languages support integration and end-to-end (E2E) testing.
JavaScript: Cypress for E2E Testing
Cypress is a popular tool for E2E testing of web applications, providing a developer-friendly interface and real-time browser interaction.
Example: Cypress Test
describe('Login Page', () => {
it('should log in with valid credentials', () => {
cy.visit('/login');
cy.get('#username').type('user');
cy.get('#password').type('password');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
});
});
Python: Selenium for Browser Automation
Selenium is commonly used in Python for E2E testing of web applications, automating browser interactions.
Example: Selenium Test
from selenium import webdriver
def test_login():
driver = webdriver.Chrome()
driver.get("http://example.com/login")
driver.find_element_by_id("username").send_keys("user")
driver.find_element_by_id("password").send_keys("password")
driver.find_element_by_css_selector("button[type='submit']").click()
assert "dashboard" in driver.current_url
driver.quit()
Key Takeaways:
- Unit Testing: JavaScript (Mocha/Chai) and Python (Pytest) frameworks are highly flexible, but Pytestโs concise syntax makes it particularly beginner-friendly.
- Test Coverage: Both
nyc
(JavaScript) andcoverage.py
(Python) are effective for measuring test coverage and identifying gaps. - E2E Testing: JavaScript developers can leverage Cypress for browser testing, while Python offers Selenium for automation.
- CI/CD Compatibility: Both languages integrate seamlessly with modern CI/CD pipelines, enabling automated testing at every stage of development.