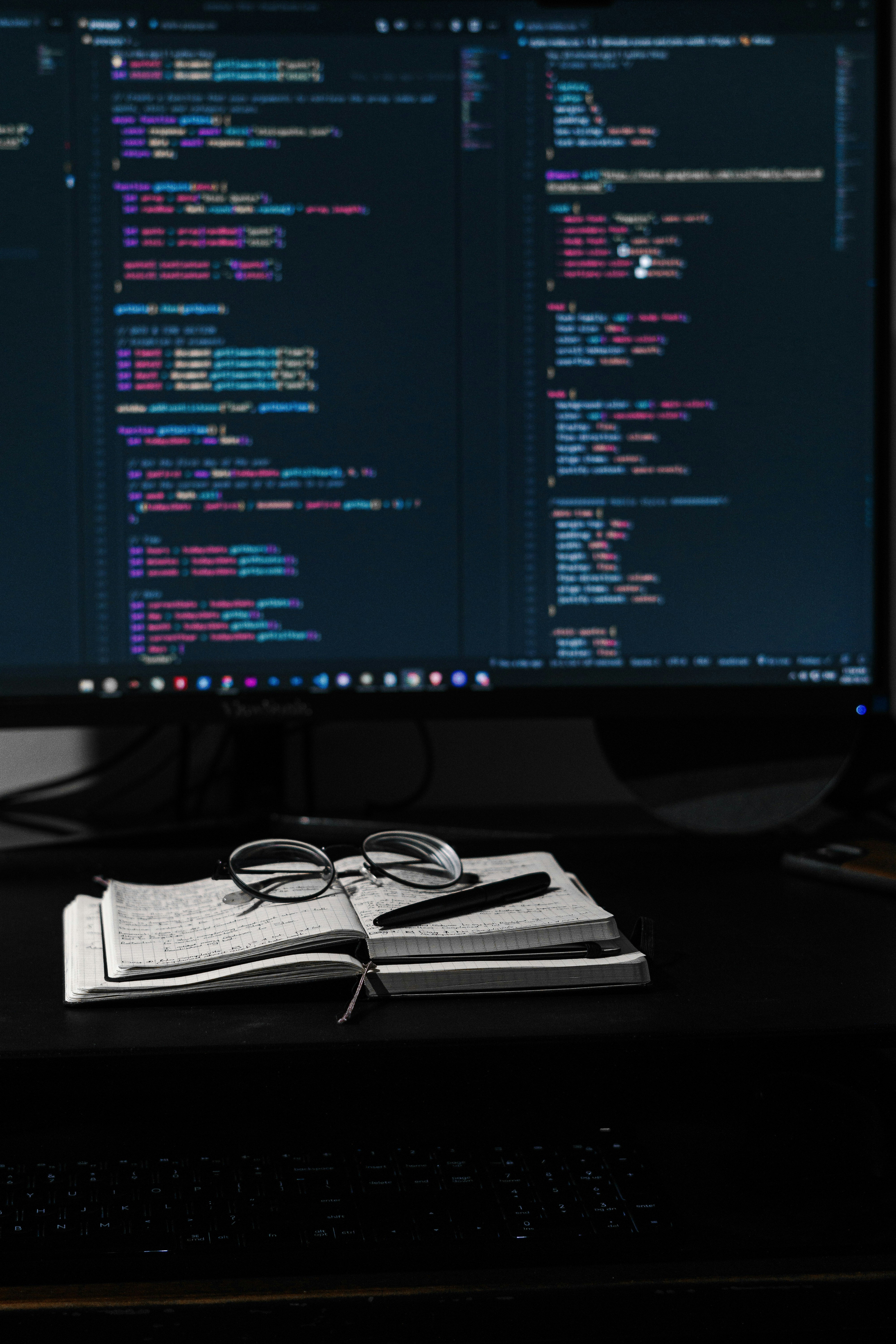
7. Asynchronous Programming
7. Asynchronous Programming 관련
Asynchronous programming is essential for handling tasks like network requests, file I/O, or any operation that takes time to complete.
Both Python and JavaScript support asynchronous programming, but their implementations differ significantly. JavaScript is inherently asynchronous and event-driven, while Python introduced asynchronous programming more recently with the asyncio
library and async/await
syntax.
Event Loop and Promises in JavaScript
JavaScript’s asynchronous model is based on the event loop, which processes tasks in a non-blocking manner. This makes it ideal for web applications where responsiveness is key. JavaScript uses callbacks, Promises, and async/await to handle asynchronous tasks.
Example: Fetching Data with Promises
A common asynchronous operation is fetching data from an API.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
How it works:
- The
fetch
function returns a Promise. - The
.then
method is used to handle the resolved Promise, whereresponse.json()
parses the JSON data. - The
.catch
method handles errors, such as network issues.
Example: Using Async/Await
Async/await simplifies the syntax for working with Promises.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
In this example, await
pauses the execution of the fetchData
function until the Promise is resolved or rejected, providing a more synchronous-like flow.
Asyncio and Await Syntax in Python
Python’s asynchronous programming revolves around the asyncio
library, which introduced the async
and await
keywords to handle asynchronous operations. Unlike JavaScript, Python does not have a built-in event loop – it relies on asyncio
to create and manage one.
Example: Fetching Data with Asyncio
Using Python’s aiohttp
library for asynchronous HTTP requests:
import asyncio
import aiohttp
async def fetch_data():
async with aiohttp.ClientSession() as session:
async with session.get('https://api.example.com/data') as response:
data = await response.json()
print(data)
asyncio.run(fetch_data())
How it works:
- The
async def
syntax defines an asynchronous function. await
is used to pause execution until theget
request completes.asyncio.run
()
starts the event loop and runs the asynchronous function.
Key Differences from JavaScript
- Python explicitly defines asynchronous functions with
async def
. - The
asyncio
library is required to run the event loop. - Python’s
async/await
syntax is more structured but requires more setup compared to JavaScript.
Use Cases and Performance Considerations
Asynchronous programming is suitable for tasks that involve waiting, such as network requests, file I/O, or database queries. Here’s how Python and JavaScript handle common use cases:
Real-Time Applications (JavaScript): JavaScript’s event-driven model makes it ideal for real-time applications like chat systems, live streaming, or collaborative tools.
Example: WebSocket in JavaScript
const socket = new WebSocket('ws://example.com/socket');
socket.onmessage = (event) => {
console.log('Message from server:', event.data);
};
I/O-Bound Tasks (Python): Python’s asynchronous model excels at handling I/O-bound tasks such as file processing, web scraping, or database queries.
Example: Asynchronous File Reading in Python
import aiofiles
import asyncio
async def read_file():
async with aiofiles.open('example.txt', mode='r') as file:
content = await file.read()
print(content)
asyncio.run(read_file())
Performance Considerations:
- Concurrency: Both languages handle concurrency well, but JavaScript’s event loop and non-blocking I/O model are better suited for high-throughput, real-time applications.
- Threading: Python’s
asyncio
works best for I/O-bound tasks. For CPU-bound tasks, Python relies on multi-threading or multi-processing. - Ease of Use: JavaScript’s async/await is simpler to implement for beginners, while Python requires familiarity with
asyncio
for similar functionality.
Key Takeaways:
- JavaScript: Asynchronous programming is central to JavaScript’s design. Its event loop and Promises make it highly efficient for real-time, event-driven applications.
- Python: Asynchronous programming is a newer addition to Python, focused on handling I/O-bound tasks efficiently with
asyncio
. - Syntax: Both languages use
async/await
, but Python requires explicit setup withasyncio
, while JavaScript integrates it natively.