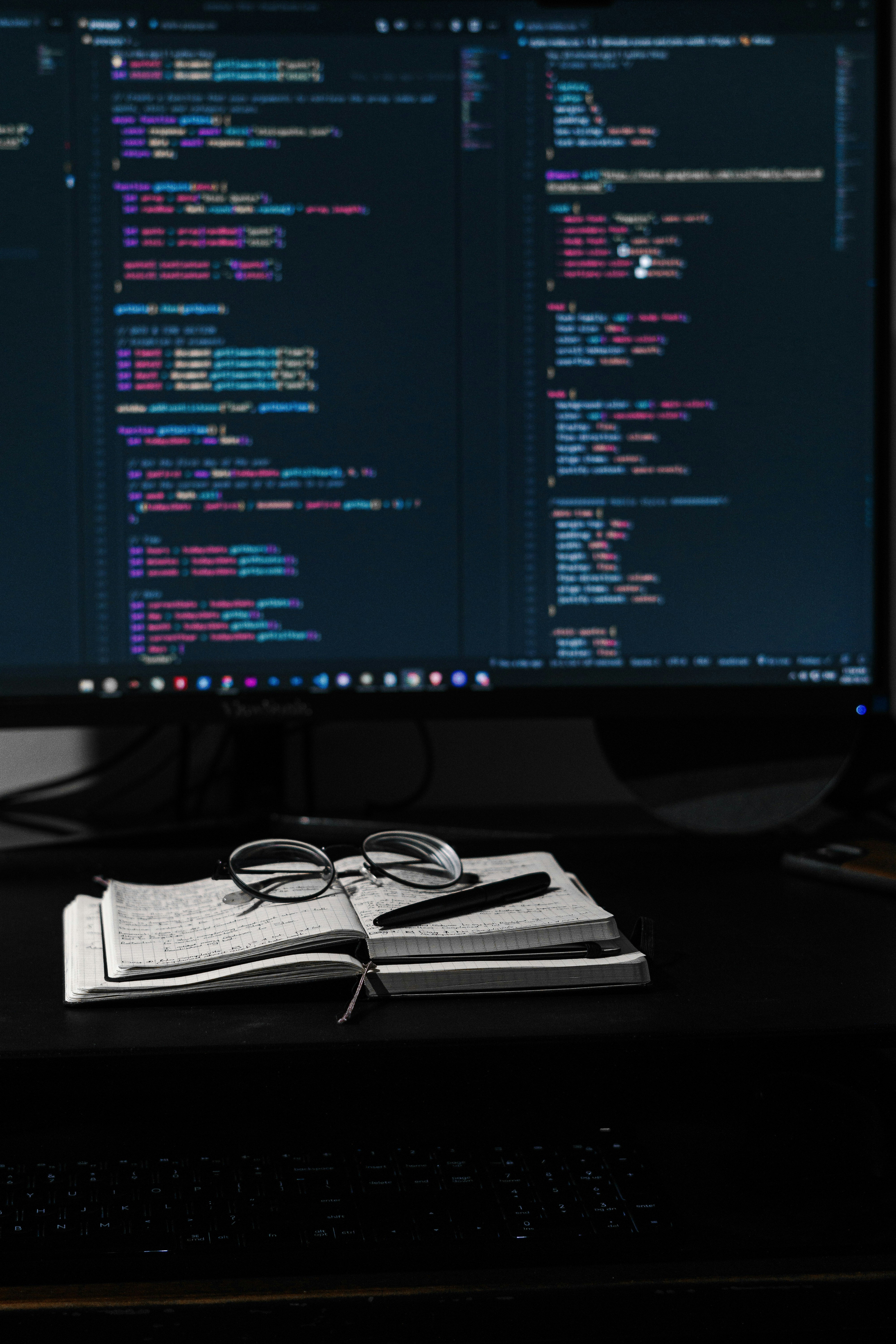
2. Language Paradigms and Use Cases
2. Language Paradigms and Use Cases 관련
JavaScript vs. Python: Scripting, Backend, and Full-Stack
Both JavaScript and Python are high-level, interpreted languages, but they were initially created with distinct purposes in mind. Over the years, they have evolved to expand their applications, making them popular choices for both general-purpose and specialized development tasks.
Understanding these differences in paradigms and common use cases helps clarify when to use each language and the kind of projects they are best suited for.
JavaScript
Known primarily as the language of the web, JavaScript was originally designed to add interactivity to HTML documents within browsers. Today, with the advent of frameworks like React, Angular, and Vue, JavaScript is at the core of modern, interactive frontend web development.
The language’s reach expanded even further with Node.js, which brought JavaScript to the server side. Now, JavaScript is a full-stack language that powers single-page applications (SPAs), RESTful APIs, and server-side rendering.
JavaScript is event-driven and asynchronous by design, making it ideal for real-time applications such as chat apps, collaborative tools, and streaming services.
Python
Initially created with a focus on readability and simplicity, Python has become one of the most versatile languages in the world. While JavaScript is often tied to web applications, Python is more commonly used in fields like scientific computing, data analysis, machine learning, and artificial intelligence. Its readability and simplicity make it a great choice for scripting, automation, and rapid prototyping.
Also, Python’s rich ecosystem of libraries and frameworks, such as Django and Flask, allow it to be used for backend web development.
Unlike JavaScript, Python is synchronous by default, which makes it better suited for tasks that don’t require real-time interaction but benefit from efficiency, such as data processing and batch operations.
Core Differences in Approach: Dynamic Typing, Functional Programming, and OOP
Both JavaScript and Python are dynamically typed, meaning that variables do not need to be declared with a specific type and can hold different types of data at runtime. But the two languages implement this dynamic typing in slightly different ways, and they each approach functional programming and object-oriented programming (OOP) differently.
Both languages allow flexibility in declaring variables without specifying types, making them highly flexible. But Python’s strict indentation requirements and clear error messages provide a more structured approach to dynamic typing.
JavaScript, on the other hand, has a looser syntax, which sometimes leads to quirks, such as type coercion, that can result in unexpected behavior (for example, 0 == ''
evaluates to true
).
Both languages support functional programming techniques, but JavaScript leans heavily on it. Functions are first-class citizens in JavaScript, allowing developers to pass functions as arguments, return them from other functions, and store them in variables. Higher-order functions, such as map
, reduce
, and filter
, are commonly used in JavaScript to process arrays and data collections.
Python also supports functional programming, and it includes a lambda
feature for anonymous functions as well as map
, filter
, and reduce
functions. But functional programming is less central in Python, which encourages readability and simplicity over deeply functional constructs.
JavaScript’s OOP model is prototype-based, meaning that objects can inherit directly from other objects without the need for classes. Since ES6, JavaScript has also included support for class
syntax, making it easier for developers coming from class-based languages to work with objects.
Python, on the other hand, uses a class-based model that is more in line with traditional OOP languages like Java and C++. Classes, inheritance, and polymorphism in Python are straightforward, making it an excellent choice for developers who prefer a clear and well-structured approach to OOP.
Typical Use Cases for JavaScript and Python
To understand the strengths of each language, it’s helpful to consider the types of projects that developers commonly use JavaScript and Python for:
Common Use Cases for JavaScript*
- Frontend Web Development: JavaScript is essential for building interactive user interfaces in web browsers. With libraries and frameworks like React, Vue, and Angular, developers can build rich, responsive applications that run entirely in the browser.
- Full-Stack Web Development: Node.js allows JavaScript to be used on the backend, enabling full-stack development with JavaScript across the entire application. Express, NestJS, and other frameworks provide the tools for creating RESTful APIs, real-time applications, and server-side rendering.
- Real-Time Applications: JavaScript’s asynchronous and non-blocking nature makes it ideal for applications that require real-time updates, such as chat applications, live streaming, and collaborative tools.
- Mobile App Development: With frameworks like React Native, JavaScript can also be used to build cross-platform mobile applications. This allows JavaScript developers to leverage their web development skills to create mobile apps that work on both iOS and Android devices.
Common Use Cases for Python*
- Data Science and Analysis: Python’s popularity in data science is unparalleled, with libraries like Pandas, NumPy, and Matplotlib providing robust tools for data manipulation, analysis, and visualization. Python is the go-to language for data scientists and analysts.
- Machine Learning and Artificial Intelligence: Python’s machine learning libraries, such as TensorFlow, Keras, and PyTorch, make it an ideal language for building machine learning models and neural networks. Python’s readability is especially useful when experimenting with complex algorithms.
- Automation and Scripting: Python’s simplicity and versatility make it a popular choice for automation. Tasks like file manipulation, batch processing, and web scraping can be accomplished with Python scripts, using libraries like BeautifulSoup, Selenium, and Requests.
- Backend Web Development: Python’s web frameworks, such as Django and Flask, provide powerful tools for creating scalable and secure web applications. Python is widely used for backend development, particularly in projects that require quick prototyping, as its concise syntax speeds up development.
- Scientific Computing and Research: Python is commonly used in scientific research due to its extensive scientific libraries, such as SciPy and SymPy, and its compatibility with environments like Jupyter notebooks.
By understanding the typical use cases and paradigms that each language supports, JavaScript developers can better appreciate Python’s unique strengths.
JavaScript is inherently event-driven, making it ideal for interactive applications, while Python’s strengths lie in readability and a well-defined structure, making it an excellent choice for projects that demand clarity and precision, like data science, scripting, and backend development.