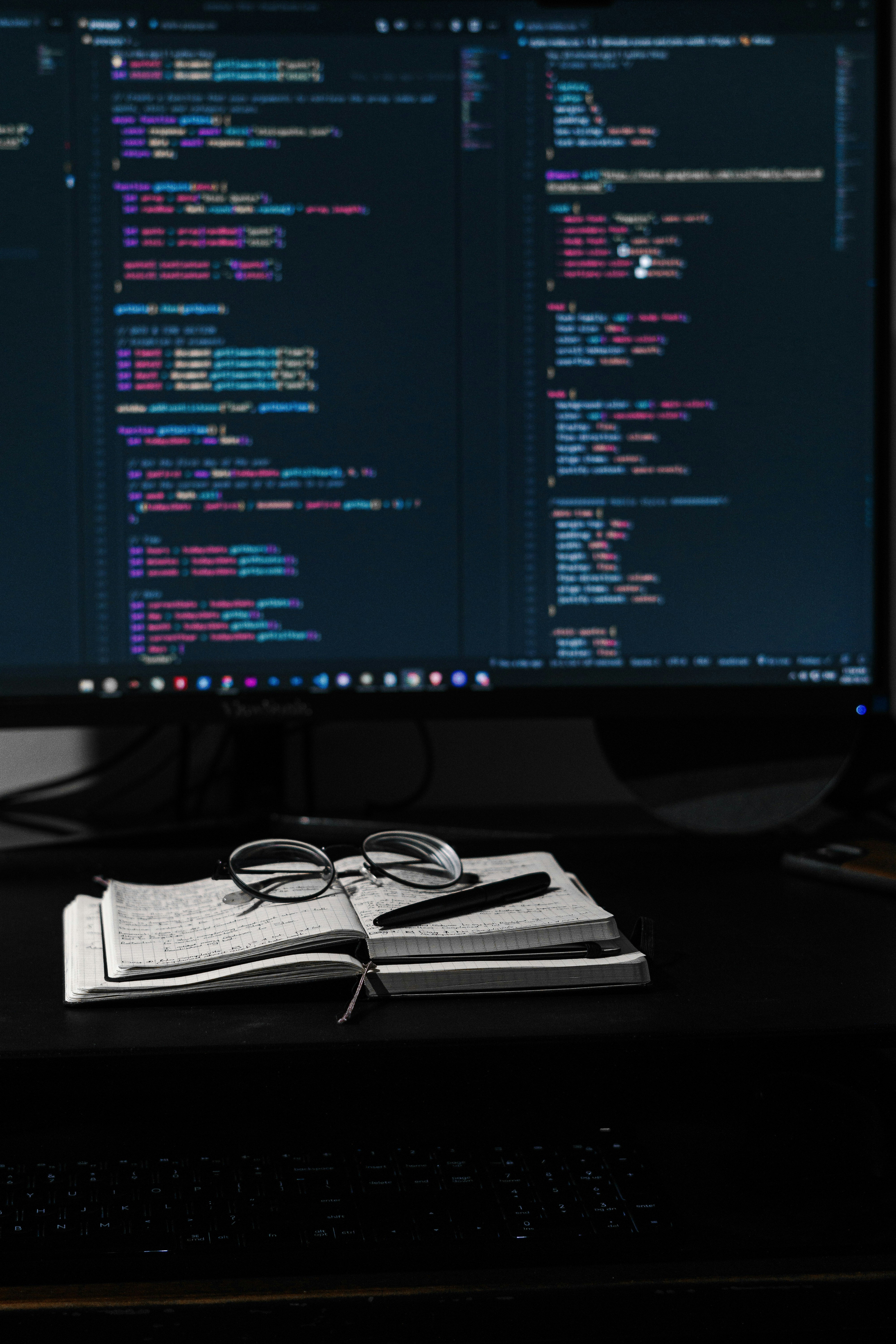
12. Community, Libraries, and Ecosystem
12. Community, Libraries, and Ecosystem ๊ด๋ จ
The strength of a programming language often lies in its community, ecosystem, and the libraries available for solving common problems. Both Python and JavaScript have vast ecosystems supported by active communities, but they cater to different domains and developer needs.
Open Source Libraries: NPM vs. PyPI
Both Python and JavaScript have centralized repositories for distributing and installing open-source libraries: PyPI (Python Package Index) for Python and NPM (Node Package Manager) for JavaScript.
Python: PyPI
- PyPI hosts over 400,000 packages, supporting fields like data science, web development, machine learning, and automation.
- Popular libraries include:
- Pandas for data manipulation.
- NumPy for numerical computing.
- Django and Flask for web development.
- BeautifulSoup and Scrapy for web scraping.
Example: Installing and Using a PyPI Library
pip install requests
import requests
response = requests.get("https://api.example.com/data")
print(response.json())
JavaScript: NPM
- NPM is the worldโs largest software registry, with over 2 million packages for frontend, backend, and full-stack development.
- Popular libraries include:
- React and Vue for frontend development.
- Express for backend services.
- Lodash for utility functions.
- Axios for HTTP requests.
Example: Installing and Using an NPM Library
npm install axios
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => console.log(response.data));
Comparison:
- Breadth: NPM focuses on web development, while PyPI covers a wider range of domains, including data science and scientific research.
- Tools: NPMโs CLI offers additional functionality like scripts and versioning, while pip focuses purely on library installation.
Key Libraries for Data Science, Web Development, and Automation
Both ecosystems excel in their respective strengths:
Data Science:
- Python dominates with libraries like Pandas, Matplotlib, and TensorFlow, making it the top choice for data manipulation, visualization, and machine learning.
- JavaScript has D3.js for interactive visualizations and TensorFlow.js for machine learning, though its ecosystem for data science is less mature.
Web Development:
- JavaScript is unrivaled in frontend development with React, Vue, and Angular. For backend services, Node.js with Express is a common choice.
- Python excels in backend web development with frameworks like Django and Flask, offering rapid development and scalability.
Automation:
- Python is widely used for scripting and automation, with libraries like
os
,shutil
, andschedule
. - JavaScript, while less focused on automation, can handle automation tasks effectively with Node.js and tools like Puppeteer for browser automation.
Python's Strengths in Data Science and Machine Learning
Python has established itself as the go-to language for data science and machine learning due to its extensive ecosystem and user-friendly syntax.
Popular Python Libraries for Data Science:
- Pandas: Data manipulation and analysis.
- NumPy: Numerical computing and arrays.
- Matplotlib/Seaborn: Data visualization.
- Scikit-learn: Machine learning algorithms.
- TensorFlow/Keras: Deep learning frameworks.
Example: Data Analysis with Pandas
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df.describe())
Machine Learning with TensorFlow
import tensorflow as tf
model = tf.keras.Sequential([tf.keras.layers.Dense(units=1, input_shape=[1])])
model.compile(optimizer='sgd', loss='mean_squared_error')
model.fit([1, 2, 3, 4], [2, 4, 6, 8], epochs=500)
print(model.predict([5]))
Pythonโs simplicity makes it easy for non-programmers, such as data analysts and researchers, to leverage these powerful tools.
JavaScript's Strengths in Web Development
JavaScriptโs dominance in web development stems from its ability to run natively in the browser and its wide array of frontend frameworks.
Popular JavaScript Libraries for Web Development:
- React: Component-based UI development.
- Vue: Simple and progressive framework for building UIs.
- Angular: Comprehensive framework for large-scale applications.
- Express: Lightweight framework for creating REST APIs.
- Next.js: Full-stack framework for React applications with server-side rendering.
Example: Creating a Frontend with React
import React from 'react';
import ReactDOM from 'react-dom';
function App() {
return <h1>Hello, World!</h1>;
}
ReactDOM.render(<App />, document.getElementById('root'));
Example: Creating a Backend with Express
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
JavaScriptโs ecosystem allows developers to build full-stack applications using a single language, streamlining development workflows.
Community Support and Contribution
Both Python and JavaScript have vibrant communities that contribute to their continuous growth and evolution:
- Python:
- Python Software Foundation (PSF) drives the language's development.
- Annual events like PyCon foster collaboration and learning.
- Strong academic adoption ensures its popularity in education and research.
- JavaScript:
- Backed by major organizations like Node.js Foundation and open-source communities.
- Events like JSConf and React Conf promote innovation.
- A highly active GitHub community ensures frequent updates and new libraries.