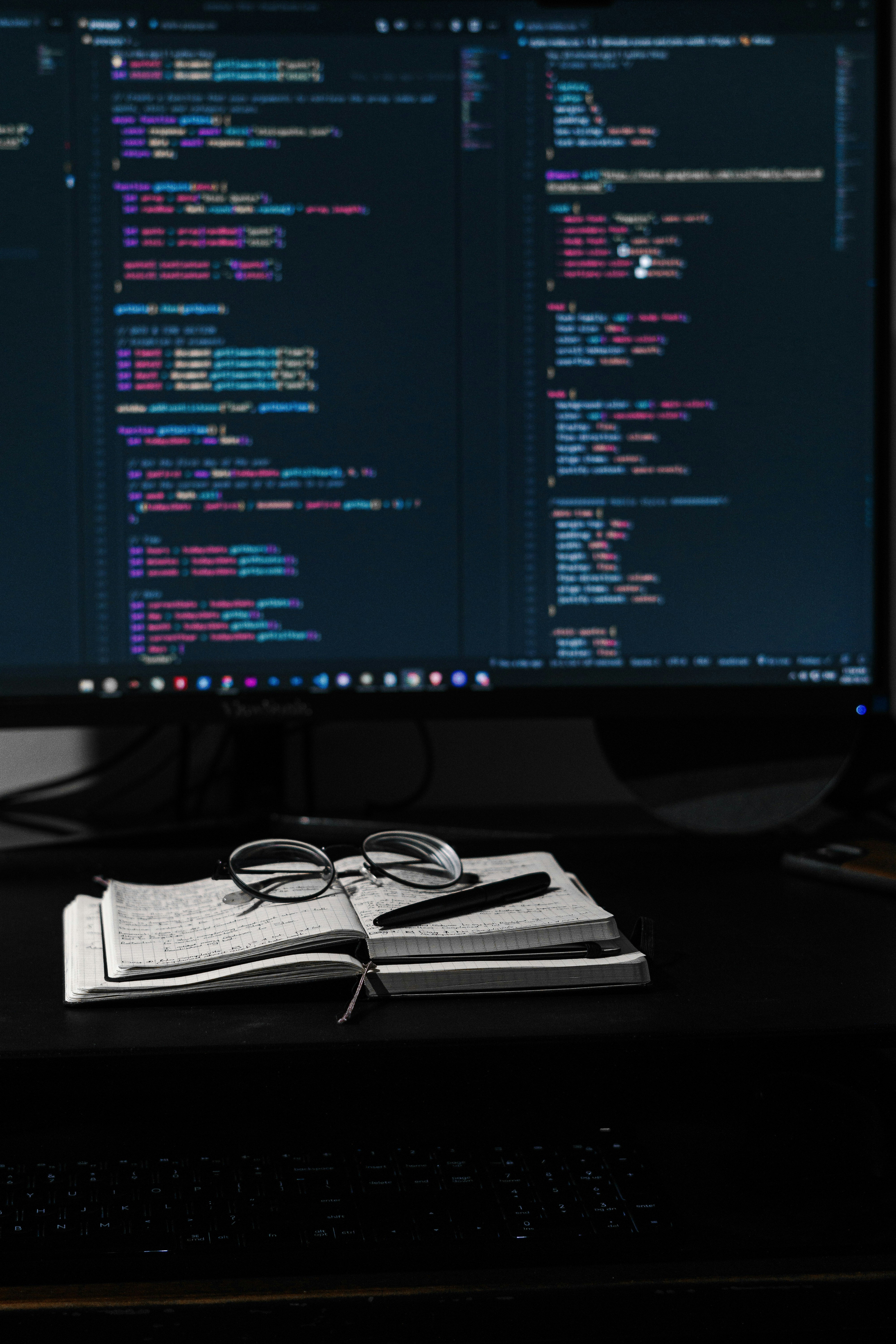
11. Practical Applications and Examples
11. Practical Applications and Examples 관련
Both Python and JavaScript excel in various practical applications, but their strengths shine in different domains. This section explores common use cases for each language, providing hands-on examples to showcase their capabilities and differences.
Writing a Simple Web Scraper
Python: Using BeautifulSoup
Python’s libraries, such as BeautifulSoup and Requests, make web scraping straightforward and efficient.
Example: Web Scraper in Python
import requests
from bs4 import BeautifulSoup
# Fetch the webpage
url = "https://example.com"
response = requests.get(url)
# Parse the HTML content
soup = BeautifulSoup(response.content, "html.parser")
# Extract specific data
titles = soup.find_all("h2")
for title in titles:
print(title.text)
JavaScript: Using Puppeteer
JavaScript can also scrape web content using libraries like Puppeteer, which allows headless browsing.
Example: Web Scraper in JavaScript
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Extract specific data
const titles = await page.$$eval('h2', elements => elements.map(el => el.textContent));
console.log(titles);
await browser.close();
})();
Key Differences:
- Python’s BeautifulSoup is simpler for static pages, while Puppeteer provides more flexibility for dynamic content rendered by JavaScript.
Creating a REST API
Python: Flask
Python’s Flask framework is lightweight and ideal for quickly building APIs.
Example: REST API in Python
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
return jsonify({"message": "Hello, World!"})
if __name__ == '__main__':
app.run(debug=True)
JavaScript: Express
Express is a popular framework for creating REST APIs in JavaScript.
Example: REST API in JavaScript
const express = require('express');
const app = express();
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello, World!' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Key Differences:
- Flask offers built-in simplicity with decorators for routing.
- Express requires more explicit configuration but is better suited for large-scale Node.js projects.
Automation Scripts: File Handling, Network Requests, and Scripting
Python: Automation with os and shutil
Python excels at automation tasks, making file and system operations straightforward.
Example: File Automation in Python
import os
import shutil
# Create a directory
os.makedirs("example_dir", exist_ok=True)
# Move a file
shutil.move("source.txt", "example_dir/destination.txt")
# List files in a directory
for file in os.listdir("example_dir"):
print(file)
JavaScript: File System Module (fs)
JavaScript’s fs
module allows file handling, but it requires more boilerplate.
Example: File Automation in JavaScript
const fs = require('fs');
const path = require('path');
// Create a directory
fs.mkdirSync('example_dir', { recursive: true });
// Move a file
fs.renameSync('source.txt', path.join('example_dir', 'destination.txt'));
// List files in a directory
fs.readdirSync('example_dir').forEach(file => {
console.log(file);
});
Key Differences:
- Python’s
os
andshutil
modules provide concise methods for file and system operations. - JavaScript requires more explicit handling for similar tasks using Node.js modules.
Data Processing and Visualization
Python: Data Science with Pandas and Matplotlib
Python dominates data processing and visualization with libraries like Pandas and Matplotlib.
Example: Data Analysis in Python
import pandas as pd
import matplotlib.pyplot as plt
# Create a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
# Plot the data
df.plot(x='Name', y='Age', kind='bar')
plt.show()
JavaScript: Data Visualization with D3.js
JavaScript excels at interactive web-based visualizations with D3.js.
Example: Data Visualization in JavaScript
const d3 = require('d3');
const data = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 }
];
const svg = d3.create("svg")
.attr("width", 500)
.attr("height", 300);
svg.selectAll("rect")
.data(data)
.enter()
.append("rect")
.attr("x", (d, i) => i * 100)
.attr("y", d => 300 - d.age * 5)
.attr("width", 50)
.attr("height", d => d.age * 5);
console.log(svg.node().outerHTML);
Key Differences:
- Python’s data libraries are geared toward analysis and are simpler for static visualizations.
- JavaScript’s D3.js creates highly interactive visualizations for web applications.
Machine Learning and AI
Python: TensorFlow
Python’s TensorFlow library simplifies building machine learning models.
Example: Machine Learning in Python
import tensorflow as tf
# Define a simple model
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=1, input_shape=[1])
])
model.compile(optimizer='sgd', loss='mean_squared_error')
# Train the model
xs = [1, 2, 3, 4]
ys = [2, 4, 6, 8]
model.fit(xs, ys, epochs=500, verbose=0)
# Predict
print(model.predict([5])) # Output: [[10]]
JavaScript: TensorFlow.js
TensorFlow.js brings machine learning capabilities to JavaScript.
Example: Machine Learning in JavaScript
const tf = require('@tensorflow/tfjs-node');
// Define a simple model
const model = tf.sequential();
model.add(tf.layers.dense({ units: 1, inputShape: [1] }));
model.compile({ optimizer: 'sgd', loss: 'meanSquaredError' });
// Train the model
const xs = tf.tensor([1, 2, 3, 4]);
const ys = tf.tensor([2, 4, 6, 8]);
model.fit(xs, ys, { epochs: 500 }).then(() => {
// Predict
model.predict(tf.tensor([5])).print(); // Output: [[10]]
});
Key Differences:
- Python dominates in machine learning due to its mature ecosystem and extensive documentation.
- TensorFlow.js allows machine learning in JavaScript, but it is less mature compared to Python’s TensorFlow.
Key Takeaways:
- Web Scraping: Python excels with BeautifulSoup for static content, while Puppeteer is better for dynamic content.
- REST APIs: Python’s Flask is lightweight and easy to use, while JavaScript’s Express offers flexibility and scalability.
- Automation: Python simplifies file and system operations with
os
andshutil
, while JavaScript achieves similar results with Node.js modules. - Data Visualization: Python’s libraries focus on analysis, while JavaScript’s D3.js creates interactive, web-based visualizations.
- Machine Learning: Python leads with TensorFlow and other ML frameworks, while TensorFlow.js brings ML capabilities to JavaScript.