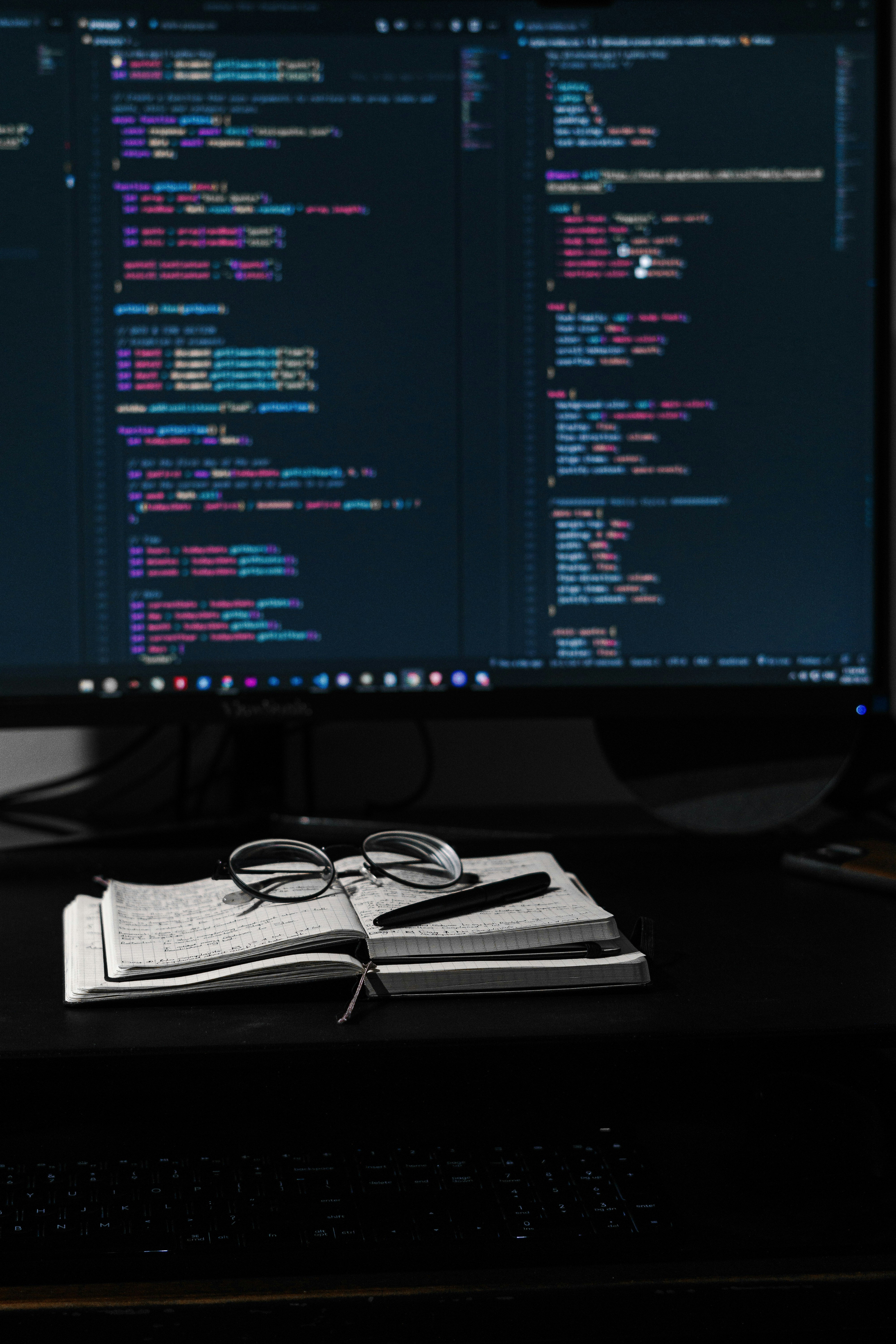
8. Modules, Packages, and Dependency Management
8. Modules, Packages, and Dependency Management κ΄λ ¨
Both Python and JavaScript encourage modular programming, allowing developers to divide code into reusable and maintainable components.
Managing modules, packages, and dependencies is essential for any non-trivial project, and both languages provide robust systems to handle these needs. But the tools and ecosystems differ significantly.
Node.js Modules vs. Python Packages
JavaScript uses the Node.js module system, which allows developers to organize code into modules. Modules can be imported using require
(CommonJS) or import
(ES6 modules).
Example: Exporting and Importing Modules in JavaScript
export function add(a, b) {
return a + b;
}
export function multiply(a, b) {
return a * b;
}
import { add, multiply } from './utils.js';
console.log(add(2, 3)); // Output: 5
console.log(multiply(2, 3)); // Output: 6
CommonJS uses module.exports
and require()
:
// utils.js
module.exports = {
add: (a, b) => a + b,
multiply: (a, b) => a * b,
};
// main.js
const { add, multiply } = require('./utils');
console.log(add(2, 3)); // Output: 5
console.log(multiply(2, 3)); // Output: 6
Python organizes reusable code into modules and packages. A module is simply a .py
file, and a package is a directory containing a special __init__.py
file, which can include one or more modules.
Example: Exporting and Importing Modules in Python
def add(a, b):
return a + b
def multiply(a, b):
return a * b
from utils import add, multiply
print(add(2, 3)) # Output: 5
print(multiply(2, 3)) # Output: 6
Python uses import
for loading modules and supports relative imports for packages.
Package Managers: NPM vs. pip
Both languages provide package managers for installing and managing third-party libraries and dependencies.
- Node Package Manager (NPM) is JavaScriptβs default package manager, and it comes bundled with Node.js.
- It uses a
package.json
file to define dependencies, scripts, and metadata for a project.
npm install express
{
"dependencies": {
"express": "^4.18.2"
}
}
- Python uses pip (Python Installer Package) to manage libraries and frameworks.
- Python projects commonly use a
requirements.txt
file to list dependencies.
pip install flask
flask==2.3.0
requests==2.31.0
To install all dependencies in requirements.txt
:
bashCopy codepip install -r requirements.txt
Comparison
- NPM allows version ranges and automatically creates
node_modules
to manage dependencies. It also supports both development (--save-dev
) and production dependencies. - pip installs libraries globally or in a virtual environment but lacks the automatic distinction between dev and production dependencies, which must be handled manually.
Managing Dependencies in Python with Virtual Environments
Python has a unique feature for isolating dependencies: virtual environments. Virtual environments ensure that dependencies for one project donβt interfere with another, avoiding conflicts.
Creating a Virtual Environment:
python -m venv myenv
Activating the Virtual Environment:
myenv\Scripts\activate
source myenv/bin/activate
Installing Libraries in the Virtual Environment:
pip install flask
Deactivating the Virtual Environment:
deactivate
JavaScript Alternative: While JavaScript does not require virtual environments, tools like nvm
(Node Version Manager) can be used to manage different Node.js versions for projects.
Project Structures and Best Practices
A typical Node.js project includes:
my-node-project/
βββ node_modules/ # Installed dependencies
βββ src/ # Source code
β βββ app.js # Entry point
β βββ utils.js # Utility module
βββ package.json # Dependency and project metadata
βββ package-lock.json # Dependency tree for consistency
my-python-project/
βββ venv/ # Virtual environment
βββ src/ # Source code
β βββ __init__.py # Package initializer
β βββ app.py # Entry point
β βββ utils.py # Utility module
βββ requirements.txt # Dependency list
Key Takeaways:
- Modules: Both languages support modular programming. Python modules are simple
.py
files, while JavaScript has both CommonJS and ES6 modules. - Package Managers: NPM and pip serve similar purposes but have different approaches. NPM is more feature-rich, supporting scripts and version management, while pip is simpler but relies on virtual environments for isolation.
- Dependency Isolation: Pythonβs virtual environments ensure clean project separation, a feature not natively required in JavaScript due to its global Node.js architecture.