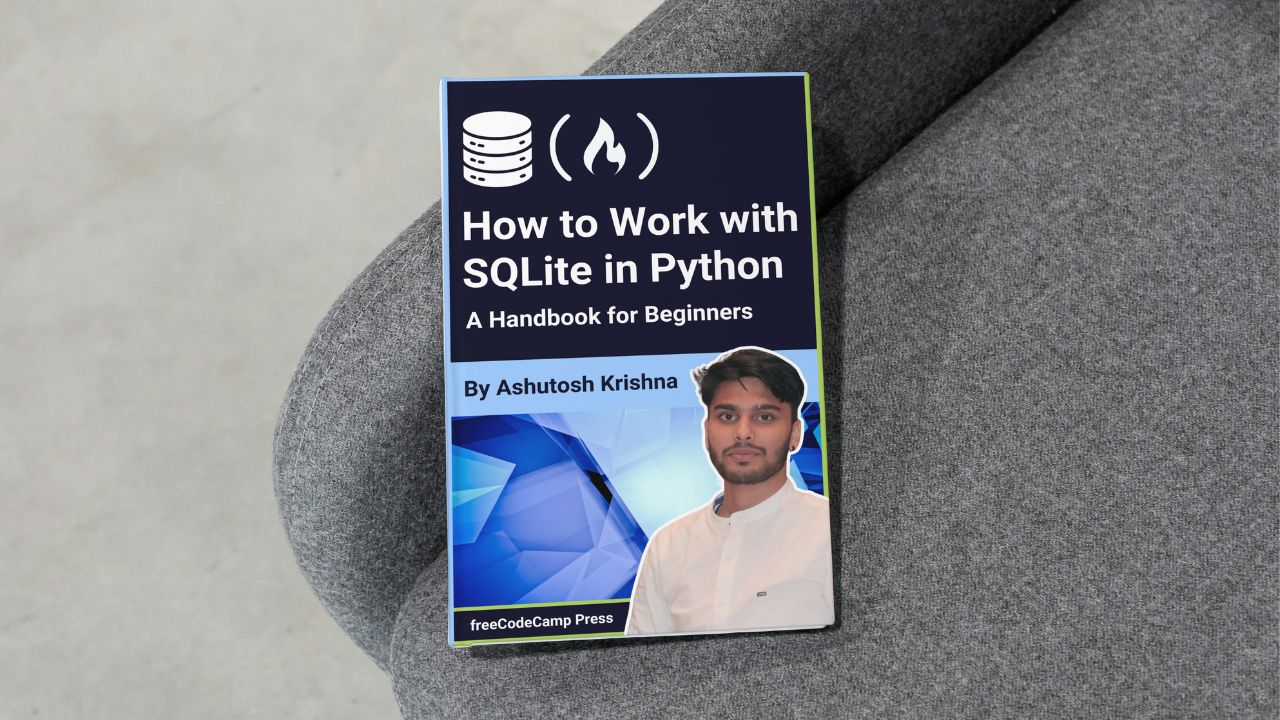
How to Handle Errors and Exceptions
October 2, 2024About 3 min
How to Handle Errors and Exceptions κ΄λ ¨
How to Work with SQLite in Python β A Handbook for Beginners
SQLite is one of the most popular relational database management systems (RDBMS). Itβs lightweight, meaning that it doesnβt take up much space on your system. One of its best features is that itβs serverless, so you donβt need to install or manage a ...
How to Work with SQLite in Python β A Handbook for Beginners
SQLite is one of the most popular relational database management systems (RDBMS). Itβs lightweight, meaning that it doesnβt take up much space on your system. One of its best features is that itβs serverless, so you donβt need to install or manage a ...
In this section, weβll discuss how to handle errors and exceptions when working with SQLite in Python. Proper error handling is crucial for maintaining the integrity of your database and ensuring that your application behaves predictably.
Common Errors in SQLite Operations
When interacting with an SQLite database, several common errors may arise:
- Constraint Violations: This occurs when you try to insert or update data that violates a database constraint, such as primary key uniqueness or foreign key constraints. For example, trying to insert a duplicate primary key will trigger an error.
- Data Type Mismatches: Attempting to insert data of the wrong type (for example, inserting a string where a number is expected) can lead to an error.
- Database Locked Errors: If a database is being written to by another process or connection, trying to access it can result in a "database is locked" error.
- Syntax Errors: Mistakes in your SQL syntax will result in errors when you try to execute your commands.
How to Use Python's Exception Handling
Pythonβs built-in exception handling mechanisms (try
and except
) are essential for managing errors in SQLite operations. By using these constructs, you can catch exceptions and respond appropriately without crashing your program.
Hereβs a basic example of how to handle errors when inserting data into the database:
import sqlite3
def add_customer_with_error_handling(name, balance):
"""Add a new customer with error handling."""
try:
with sqlite3.connect('my_database.db') as connection:
cursor = connection.cursor()
cursor.execute(
"INSERT INTO Customers (name, balance) VALUES (?, ?);", (name, balance))
connection.commit()
print(f"Added customer: {name} with balance: {balance}")
except sqlite3.IntegrityError as e:
print(f"Error: Integrity constraint violated - {e}")
except sqlite3.OperationalError as e:
print(f"Error: Operational issue - {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
# Example usage
add_customer_with_error_handling('Vishakha', 100.0) # Valid
add_customer_with_error_handling('Vishakha', 150.0) # Duplicate entry
In this example:
- We catch
IntegrityError
, which is raised for violations like unique constraints. - We catch
OperationalError
for general database-related issues (like database locked errors). - We also have a generic
except
block to handle any unexpected exceptions.
Output:
Added customer: Vishakha with balance: 100.0
Error: Integrity constraint violated - UNIQUE constraint failed: Customers.name
Best Practices for Ensuring Database Integrity
- Use Transactions: Always use transactions (as discussed in the previous section) when performing multiple related operations. This helps ensure that either all operations succeed or none do, maintaining consistency.
- Validate Input Data: Before executing SQL commands, validate the input data to ensure it meets the expected criteria (for example, correct types, within allowable ranges).
- Catch Specific Exceptions: Always catch specific exceptions to handle different types of errors appropriately. This allows for clearer error handling and debugging.
- Log Errors: Instead of just printing errors to the console, consider logging them to a file or monitoring system. This will help you track issues in production.
- Graceful Degradation: Design your application to handle errors gracefully. If an operation fails, provide meaningful feedback to the user rather than crashing the application.
- Regularly Backup Data: Regularly back up your database to prevent data loss in case of critical failures or corruption.
- Use Prepared Statements: Prepared statements help prevent SQL injection attacks and can also provide better performance for repeated queries.