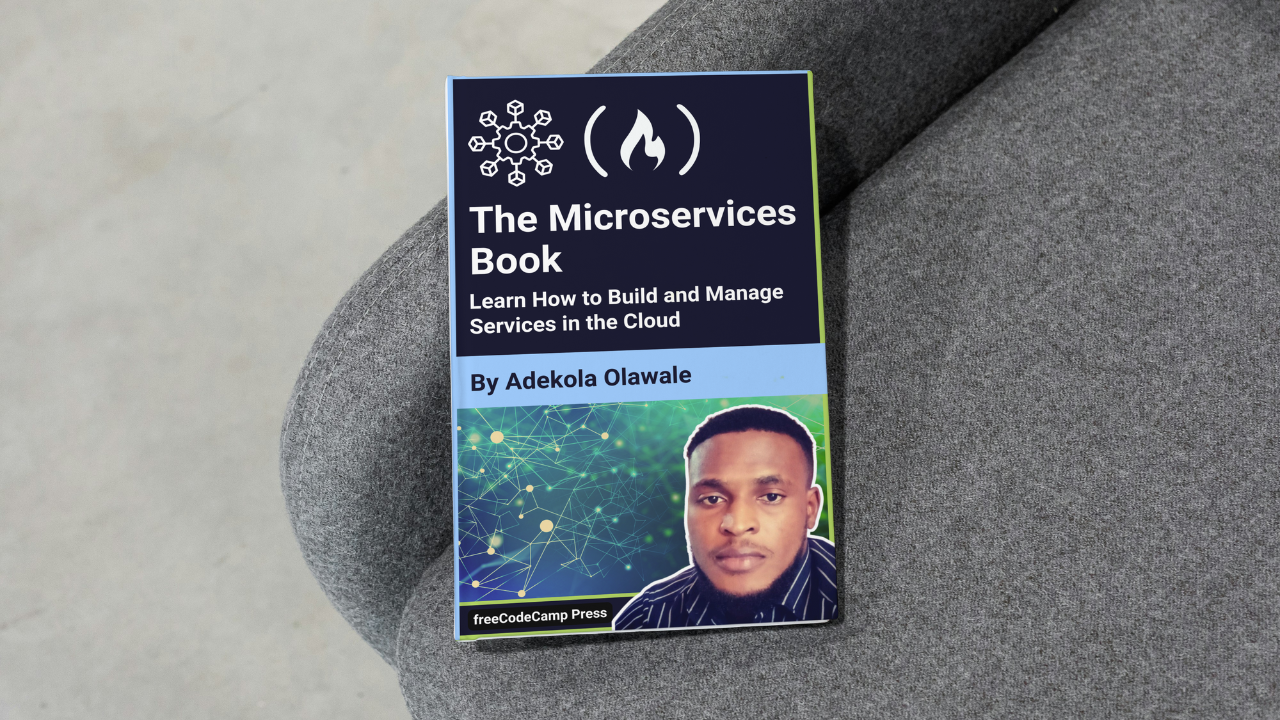
Case Studies and Real-World Examples
Case Studies and Real-World Examples 관련
The section explores how microservices architecture has been implemented across various industries, offering insights into the successes, challenges, and innovations from leading companies.
By examining real-world applications, you’ll see how microservices are used to solve complex scalability and flexibility issues and how different companies have approached architecture, deployment, and management.
This section includes detailed case studies from technology giants and enterprises in sectors such as e-commerce, finance, and media, showcasing how each adapted microservices to meet unique demands.
By analyzing both the strategies that drove successful implementations and the lessons learned from obstacles encountered, this part provides a practical perspective on microservices adoption and illustrates how abstract concepts are applied in real-world environments.
Through these examples, you should be able to grasp how microservices might benefit your own applications, gaining actionable insights for building, scaling, and optimizing microservices in diverse operational contexts.
Case Study 1: E-Commerce Platform
First, we’ll look at the case of an e-commerce platform with multiple microservices handling product listings, user management, order processing, and payment transactions.
Think of the platform as a large department store with separate sections for clothing, electronics, and groceries. Each section (microservice) manages its own inventory and operations.
Microservices involved:
- Product Service: Manages product catalog and search functionality.
- User Service: Handles user registration, authentication, and profile management.
- Order Service: Processes orders and manages order history.
- Payment Service: Handles payment processing and transactions.
// Service Definitions
// Product Service
class ProductService {
constructor() {
this.products = [];
}
addProduct(product) {
this.products.push(product);
return product;
}
searchProducts(query) {
return this.products.filter(p => p.name.includes(query));
}
}
// User Service
class UserService {
constructor() {
this.users = [];
}
registerUser(user) {
this.users.push(user);
return user;
}
authenticateUser(username, password) {
return this.users.find(u => u.username === username && u.password === password);
}
}
// Order Service
class OrderService {
constructor() {
this.orders = [];
}
createOrder(order) {
this.orders.push(order);
return order;
}
getOrder(orderId) {
return this.orders.find(o => o.id === orderId);
}
}
// Payment Service
class PaymentService {
processPayment(paymentInfo) {
// Simulate payment processing
return `Payment of ${paymentInfo.amount} processed successfully`;
}
}
The code above illustrates how each of the four services in a microservices-oriented application is defined independently, with dedicated methods for handling distinct functionalities related to products, users, orders, and payments.
This approach exemplifies how each service in a microservice architecture is specialized and modular, with minimal dependencies on other services, which makes the codebase easier to manage, test, and scale.
The ProductService
class manages a list of products, providing methods like addProduct
to add a product to the list and searchProducts
to filter products based on a search query. The addProduct
method appends a new product to an array, simulating a lightweight in-memory data store.
The searchProducts
method then allows users to search for products by name, providing a simple but effective mechanism for retrieving relevant products based on the user’s input.
The UserService
class represents the logic for handling user-related operations. It includes a registerUser
method to add new users to the system, and an authenticateUser
method to validate credentials.
When a user attempts to log in, authenticateUser
checks for a user entry that matches both the provided username and password, simulating a basic form of user authentication.
This demonstrates how user authentication can be encapsulated within a single service, ensuring the functionality is cohesive and logically separated from other service responsibilities.
The OrderService
class is focused on managing orders. The createOrder
method allows for creating a new order, appending it to the orders
array, and returning the created order as confirmation.
The getOrder
method retrieves a specific order based on its ID, offering a way to access individual order details. This separation of concerns keeps the order-handling logic contained within its own service, making it easy to scale independently as order volumes increase.
Finally, the PaymentService
class provides a processPayment
method to simulate payment processing. This method takes payment information, such as an amount, and returns a confirmation message to indicate successful processing.
Although the processPayment
method here is simple, in a real-world scenario, it would interact with external payment processing systems. By isolating payment logic in its own service, it becomes straightforward to modify or replace the payment processing mechanism without affecting other parts of the application.
This setup demonstrates how each service can independently perform its designated tasks, enabling scalable and maintainable code. Each service manages its own state and operations without interfering with others, allowing for independent development, testing, and deployment of each service, which is a key benefit of microservice architecture.
- Challenge: Ensuring consistent data across services, such as synchronizing user data with orders.
- Solution: Implementing a shared data store or using event-driven architecture to keep data in sync.
It’s like having a central inventory system that updates stock levels across all departments in real time.
- Scalability: Separating services allowed the platform to scale individual components (for example, product search) based on demand.
- Resilience: Microservices architecture improved fault tolerance. If one service failed, the rest continued to operate.
Case Study 2: Streaming Media Service
The next case we’ll look at is a streaming service providing video content with features like recommendation engines, user profiles, and content delivery.
It’s similar to a cable TV provider with different channels (services) for live TV, on-demand content, and user recommendations.
Microservices involved:
- Content Service: Manages video content and metadata.
- Recommendation Service: Provides personalized content recommendations based on user behavior.
- User Profile Service: Handles user profiles, preferences, and watch history.
- Streaming Service: Manages video streaming and delivery.
// Service Definitions
// Content Service
class ContentService {
constructor() {
this.contents = [];
}
addContent(content) {
this.contents.push(content);
return content;
}
getContent(id) {
return this.contents.find(c => c.id === id);
}
}
// Recommendation Service
class RecommendationService {
constructor() {
this.recommendations = {};
}
generateRecommendations(userId) {
// Simulate recommendation logic
return this.recommendations[userId] || [];
}
}
// User Profile Service
class UserProfileService {
constructor() {
this.profiles = [];
}
getUserProfile(userId) {
return this.profiles.find(p => p.userId === userId);
}
}
// Streaming Service
class StreamingService {
streamContent(contentId) {
return `Streaming content with ID: ${contentId}`;
}
}
In the code above, you can see how each service encapsulates specific functionalities related to content management, user recommendations, user profiles, and streaming, typical in a media platform with a microservices architecture.
Each service class represents a distinct part of the application, ensuring modularity and separation of concerns, which aligns with the microservice philosophy.
The ContentService
class is designed to manage content data. It contains an array, this.contents
, which acts as a temporary in-memory storage for content objects. The addContent
method allows new content to be added to this array and returns the added content, allowing confirmation of a successful addition.
The getContent
method retrieves a specific content item by ID, simulating a database search. In this code, you can see how addContent
and getContent
work to handle basic content management within a defined scope, enabling simple CRUD (Create, Read, Update, Delete) operations that could later expand with a persistent data store.
The RecommendationService
class focuses on providing content recommendations based on user IDs. Here, this.recommendations
is an object where recommendations for each user can be stored and accessed.
The generateRecommendations
method fetches recommendations for a given userId
, providing a placeholder for more sophisticated recommendation logic, such as algorithms that analyze user preferences or historical data.
Also, you can see how generateRecommendations
works to encapsulate user-specific recommendations, allowing for customization and personalization of content, which is crucial for engagement in media services.
The UserProfileService
class manages user profile data. The getUserProfile
method retrieves a specific user profile based on userId
, making it possible to access user-specific information like preferences or watch history.
This service has its own in-memory array, this.profiles
, which represents user profile storage. In this code, you can see how getUserProfile
works independently to fetch relevant profile information without relying on other services, allowing it to operate autonomously and at scale.
Lastly, the StreamingService
class is responsible for handling content streaming. It includes the streamContent
method, which takes a contentId
and simulates streaming functionality by returning a message confirming the stream of the specified content.
This class doesn’t maintain state but performs an action based on a request, making it lightweight and efficient for handling multiple streaming requests. You can also see how streamContent
works by focusing solely on providing a streaming response, aligning with the principle of single responsibility and ensuring that streaming functionality remains isolated from other application logic.
These services illustrate how dividing an application into focused, specialized services allows each to operate independently. Each service’s methods are designed to be extensible, meaning they can grow in functionality without interfering with other parts of the application.
This architecture is highly advantageous for complex applications, as it allows for individual services to be scaled, modified, and maintained without impacting the overall system.
- Challenge: Handling high traffic and ensuring smooth streaming during peak times.
- Solution: Implementing content delivery networks (CDNs) and optimizing streaming protocols.
It’s like distributing TV signals through multiple antennas to ensure clear reception even in high-demand areas.
- Performance: CDN integration improved content delivery speed and reduced latency.
- Personalization: Personalized recommendations increased user engagement and satisfaction.
Case Study 3: Financial Services Application
For our third case study, we’ll consider a financial services application with microservices for account management, transaction processing, and fraud detection.
it’s similar to a bank with different departments for account services, transaction handling, and security checks.
Microservices involved:
- Account Service: Manages user accounts and balances.
- Transaction Service: Handles transactions and transfers.
- Fraud Detection Service: Monitors and detects suspicious activities.
// Service Definitions
// Account Service
class AccountService {
constructor() {
this.accounts = [];
}
createAccount(account) {
this.accounts.push(account);
return account;
}
getAccount(accountId) {
return this.accounts.find(a => a.id === accountId);
}
}
// Transaction Service
class TransactionService {
constructor() {
this.transactions = [];
}
processTransaction(transaction) {
this.transactions.push(transaction);
return transaction;
}
}
// Fraud Detection Service
class FraudDetectionService {
detectFraud(transaction) {
// Simulate fraud detection
if (transaction.amount > 10000) {
return 'Suspicious transaction detected';
}
return 'Transaction is safe';
}
}
Here, the code illustrates how each class represents a specific service within a financial application, reflecting the modular approach of a microservices architecture.
Each service focuses on a single aspect of the financial domain—account management, transaction handling, and fraud detection—ensuring the code remains organized, reusable, and scalable as each class can operate independently.
The AccountService
class is responsible for managing user accounts. Within the constructor, this.accounts
is initialized as an empty array to serve as temporary in-memory storage for account objects.
The createAccount
method allows new accounts to be created and added to the accounts
array, returning the created account for verification or further use. The getAccount
method searches through this.accounts
to find an account that matches a specific accountId
. In this code, you can see how createAccount
and getAccount
work together to provide basic CRUD operations for managing account data.
The TransactionService
class focuses on processing and recording transactions. The this.transactions
array is set up within the constructor to store individual transaction records. The processTransaction
method receives a transaction object, adds it to the transactions array, and returns it, simulating a simple method to store and track transactions.
Further in the code, you can see how processTransaction
works as a core feature of this service, facilitating transaction management independently from other services like fraud detection or account management.
The FraudDetectionService
class is built to monitor transactions for potential fraud. It includes a single method, detectFraud
, that evaluates a given transaction object based on a simple rule: if the transaction amount exceeds $10,000, it is considered “suspicious.” If the amount is less than or equal to $10,000, it is classified as “safe.”
While this is a basic example, it demonstrates how logic specific to fraud detection can be encapsulated within its own service, allowing for future expansion or integration with advanced fraud detection algorithms. You can also see how detectFraud
works to isolate and centralize fraud detection logic, making it easy to refine this logic independently as requirements evolve.
Overall, this setup illustrates how microservices can enhance modularity by separating concerns and isolating different areas of functionality. Each class has its specific responsibilities, ensuring that each service can be developed, scaled, or maintained independently without affecting the others.
This approach aligns well with a microservices architecture, as it supports scalability, code reusability, and ease of testing, allowing each service to evolve alongside the needs of the application.
- Challenge: Ensuring security and compliance with financial regulations.
- Solution: Implementing robust encryption, secure authentication mechanisms, and regular audits.
It’s like having a secure vault and stringent checks to protect and verify financial transactions.
- Security: Advanced fraud detection algorithms improved the system's ability to identify and prevent fraudulent transactions.
- Compliance: Regular updates and compliance checks ensured adherence to financial regulations.