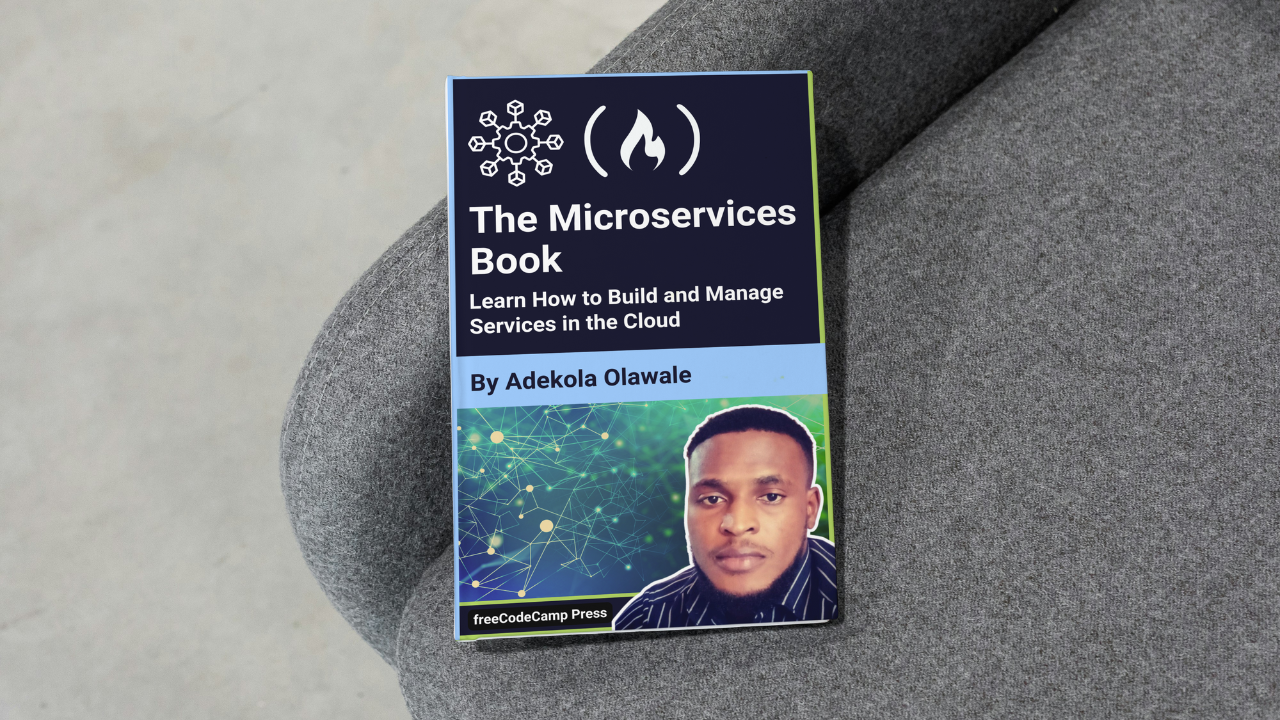
Security Considerations
Security Considerations κ΄λ ¨
Securing APIs and Inter-Service Communication (OAuth, JWT)
- OAuth 2.0: A framework that allows users to grant third-party applications access to their resources without sharing credentials.
- JWT (JSON Web Tokens): Used for secure, stateless authentication between services.
Securing API with JWT in Node.js
const jwt = require('jsonwebtoken');
// Middleware to verify JWT
function verifyToken(req, res, next) {
const token = req.headers['authorization'];
if (!token) return res.status(403).send('No token provided.');
jwt.verify(token, 'secretkey', (err, decoded) => {
if (err) return res.status(500).send('Failed to authenticate token.');
req.userId = decoded.id;
next();
});
}
app.use(verifyToken);
In this implementation, youβll notice how JWT (JSON Web Token) authentication is implemented in a Node.js application using the jsonwebtoken
library to secure API access. JWT is commonly used to verify the identity of a user and ensure that only authenticated users can access certain endpoints or perform sensitive actions.
Here, a middleware function verifyToken
is defined to check the presence and validity of a JWT token on each request. In Node.js applications, middleware is a function that has access to the request (req
) and response (res
) objects and can perform operations before passing control to the next middleware or route handler.
By setting up this middleware, you enforce token verification on every request, ensuring that all subsequent routes are protected.
The verifyToken
function first checks for a token in the request headers under the authorization
field. If no token is provided, it immediately returns a 403
status with a message indicating "No token provided," blocking access to unauthorized users.
If a token is present, the function uses jwt.verify()
to decode and validate the token against a secret key, here referred to as 'secretkey'
. If the token verification fails (for example, if the token is expired or has been tampered with), an error is returned with a 500
status code and a message indicating "Failed to authenticate token."
If the token is valid, the decoded tokenβs id
(which could represent the user's ID or other identifying information) is assigned to req.userId
, making it available for any downstream functions to use, and the next()
function is called to proceed to the next middleware or route handler.
Finally, app.use(verifyToken);
applies this middleware globally to all routes, meaning every incoming request to the API will go through this authentication check. This setup is useful in securing sensitive routes, as it prevents unauthorized users from accessing data or functionalities they shouldnβt have access to.
With this structure, you can also customize the JWT verification process or apply this middleware selectively to specific routes depending on the security requirements of your application.
Network Security and Firewall Configurations
Securing the network layer involves setting up firewall rules, VPNs, and Virtual Private Clouds (VPCs) to control access between services.
Example
Configure AWS Security Groups to restrict access to a microservice only from specific IP addresses or other services.
Compliance and Data Protection (GDPR, HIPAA)
Microservices handling sensitive data must comply with data protection regulations like GDPR (General Data Protection Regulation) and HIPAA (Health Insurance Portability and Accountability Act). This involves:
- Data encryption (in transit and at rest).
- Role-based access control (RBAC).
- Regular auditing and reporting.
Managing microservices in the cloud requires leveraging cloud-native tools, container orchestration, CI/CD practices, monitoring, and security measures.
By implementing these strategies, microservices can be deployed and managed effectively in the cloud environment while ensuring reliability, scalability, and security.