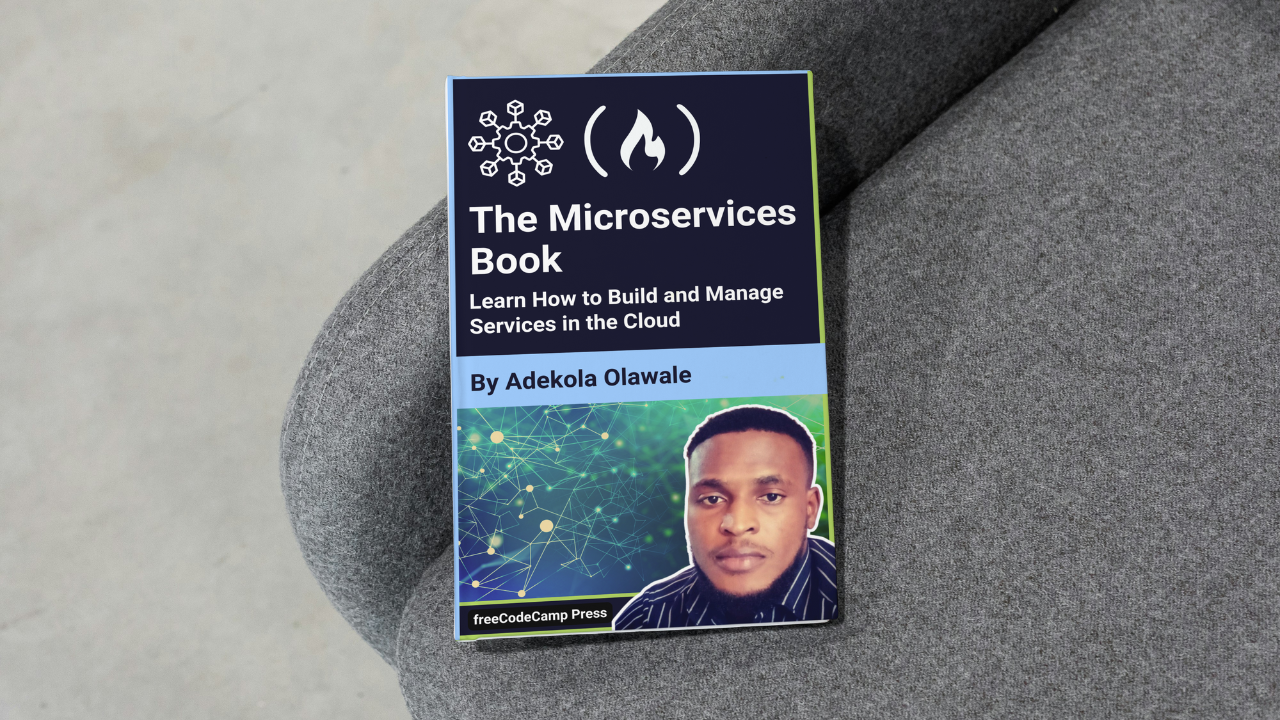
Service Discovery and Load Balancing
Service Discovery and Load Balancing κ΄λ ¨
Service Discovery Mechanisms
Service discovery mechanisms help you automatically locate and interact with services in a distributed system.
Itβs like a company directory where employees can find the contact details of their colleagues.
// Simulated service discovery using a mock service discovery
const services = {
userService: 'http://localhost:3001',
orderService: 'http://localhost:3002'
};
function getServiceUrl(serviceName) {
return services[serviceName];
}
console.log('User Service URL:', getServiceUrl('userService'));
In this code, you can see how service discovery is implemented with a simple lookup structure:
- Service Directory (Mock Service Discovery): The
services
object acts as a mock directory that maps service names (likeuserService
andorderService
) to their URLs (for example,http://localhost:3001
for the User Service). In real-world applications, this directory would be managed by a dedicated service discovery tool (such as Consul, Eureka, or etcd) rather than a static object. These tools keep track of available service instances and their locations, handling updates when services start or stop. - Dynamic URL Resolution: The
getServiceUrl
function accepts a service name as an argument and returns the corresponding URL by looking it up in theservices
directory. Here, the codegetServiceUrl('userService')
returnshttp://localhost:3001
. This allows a client or another service to dynamically resolve and access the URL foruserService
, decoupling the services by avoiding hardcoded URLs. - Example Output: The final
console.log
line demonstrates fetching the User Service URL using thegetServiceUrl
function, allowing dynamic access. The returned URL can be used by other services to make HTTP requests to the User Service.
The analogy here is like using a company directory to look up a colleague's contact details rather than remembering each individualβs location or number.
In a microservices architecture, service discovery mechanisms like this make the system more resilient and flexible, as services can be added, removed, or scaled without directly impacting other services that depend on them.
Load Balancing Strategies
Load balancing involves distributing network traffic across multiple servers to ensure efficient use of resources.
Itβs like a traffic light that directs cars to different lanes to manage traffic flow.
// Simulated load balancing
const servers = ['http://localhost:3001', 'http://localhost:3002'];
function getServer() {
return servers[Math.floor(Math.random() * servers.length)];
}
console.log('Selected Server:', getServer());
In the code above, you can see how load balancing is simulated using an array of server URLs and a simple randomization technique:
- Server Pool: The
servers
array contains a list of URLs representing different servers or instances of the same service (for example, two instances of a web application running on different ports,http://localhost:3001
andhttp://localhost:3002
). In a production environment, this list would typically include the actual IP addresses or URLs of servers that can handle the load. - Random Load Balancing Strategy: The
getServer
function picks a server at random by selecting an index within theservers
array. It generates a random number usingMath.random()
and multiplies it by the length of theservers
array. Then,Math.floor()
rounds this value down to the nearest whole number, ensuring it corresponds to a valid index in theservers
array. This strategy simulates random load balancing by choosing one server for each request, which can help distribute requests fairly evenly in smaller setups. - Output: Finally,
console.log('Selected Server:', getServer());
demonstrates which server was selected. Each timegetServer()
is called, it may pick a different server, showing how incoming requests would be balanced across the available options.
In real-world scenarios, load balancers often use more sophisticated strategies, such as round-robin (cycling through servers in sequence) or least connections (sending traffic to the server with the fewest active connections).
The analogy here is like a traffic light directing cars into different lanes: each lane is a server, and the traffic light (load balancer) distributes vehicles (requests) to prevent congestion.
This simple load-balancing code illustrates the concept of spreading requests across servers, which can improve performance and system resilience by reducing the chances of overloading any single server.