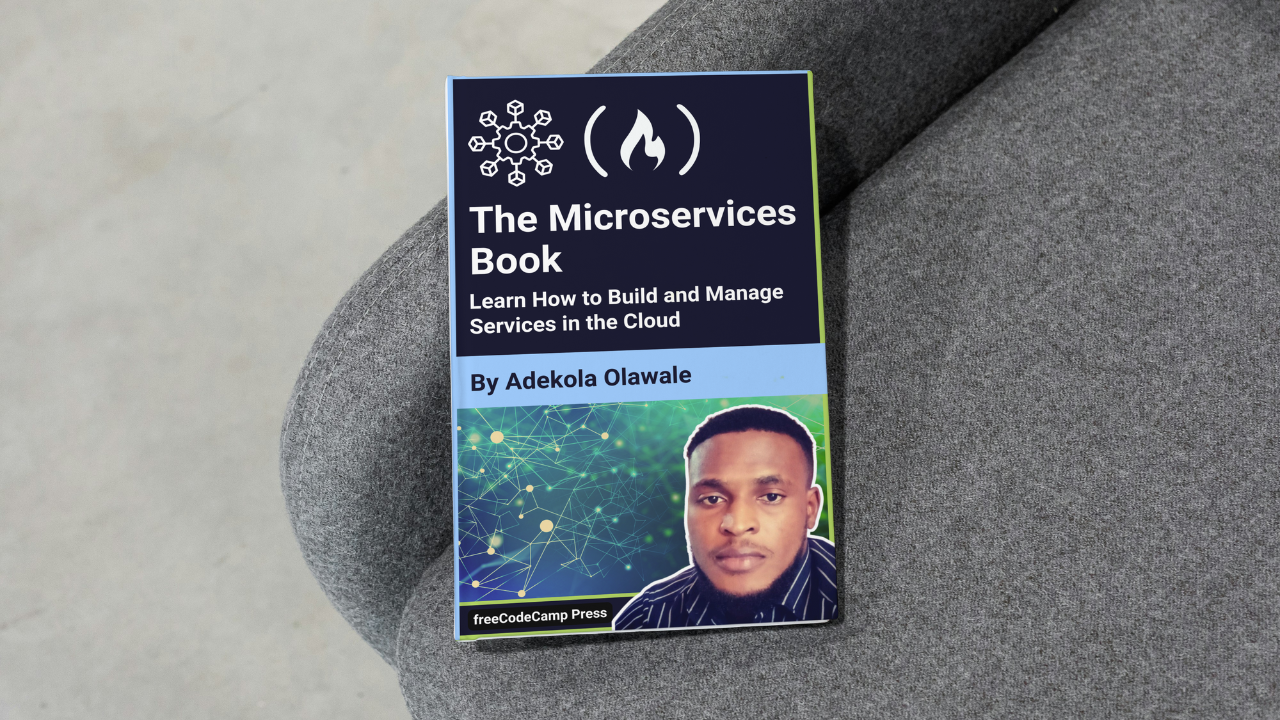
Future Trends and Innovations
Future Trends and Innovations 관련
In this section, we will discuss some cutting-edge developments and emerging trends that are shaping the future of microservices architecture. This section will examine the impact of new technologies and methodologies, such as serverless computing, micro frontends, and the use of AI-driven automation in service orchestration and management.
We’ll also look at the evolving role of DevOps and continuous integration/continuous delivery (CI/CD) pipelines in enhancing microservices deployment and maintenance.
Then we’ll discuss advancements in service mesh technologies, the increasing importance of observability and monitoring tools, and the rise of event-driven architecture as a complement to traditional request-response communication in microservices.
By the end of this section, you’ll gain insights into how these innovations are pushing microservices architecture forward, helping organizations further streamline, scale, and optimize their applications.
This forward-looking view will equip you with knowledge on potential tools and strategies that can keep your applications competitive and adaptable in a rapidly changing technological landscape.
Serverless Architecture
Serverless architecture allows you to build and run applications without managing servers.
Functions are executed in response to events, and resources are automatically scaled based on demand.
Imagine a coffee shop where you order coffee through an app. The coffee shop only needs to prepare coffee when an order is placed, and you don’t need to worry about the kitchen staff or equipment.
AWS Lambda Function
// Example of an AWS Lambda function
exports.handler = async (event) => {
console.log('Event received:', event);
// Process the event and return a response
return {
statusCode: 200,
body: JSON.stringify({ message: 'Hello from Lambda!' }),
};
};
This code depicts how an AWS Lambda function is defined to handle and process events. AWS Lambda is a serverless compute service that allows you to run code without provisioning or managing servers.
In this code example, the function is set up to run in response to an event—whether that’s an HTTP request, an update in a data source, or any other event that can trigger a Lambda function.
The function's entry point is the exports.handler
, which is structured as an asynchronous function with an event
parameter. This event
parameter contains the data relevant to the trigger, like request details if invoked through API Gateway or object information if triggered by S3. The console.log('Event received:', event);
line logs the event data to AWS CloudWatch, which is useful for debugging and tracking the input data Lambda received. This log output helps monitor and troubleshoot the function's operation and behavior by examining the event data and ensuring it is processed as expected.
Following the logging statement, the code returns a response object. Here, it returns an object with statusCode
set to 200
, indicating a successful request, and a body
field containing a JSON stringified message. This JSON message ({ message: 'Hello from Lambda!' }
) is typical for RESTful APIs and provides a response payload that a client can interpret.
The statusCode
and body
fields are crucial when the Lambda function is integrated with API Gateway, as they enable Lambda to respond to HTTP requests in a format that is directly consumable by web clients or applications.
This example shows how Lambda functions can perform a wide range of tasks triggered by various events, making them suitable for microservices and scalable cloud applications where functions execute code only when invoked, minimizing costs and resource usage.
The use of asynchronous processing (async
) allows the function to handle any potential network or data-fetching tasks non-blockingly, which is ideal for serverless environments where efficiency and quick execution are prioritized.
- Benefits: Reduced infrastructure management, automatic scaling, and pay-per-use pricing.
- Challenges: Cold start latency, limited execution time, and complexity in debugging and monitoring.
It’s like ordering takeout from a restaurant—convenient and flexible, but you rely on the restaurant’s setup and might have to wait if they’re busy.
- Improved Cold Start Times: Techniques to reduce latency for serverless functions.
- Enhanced Monitoring and Debugging: Better tools for tracking and debugging serverless applications.
Service Meshes
A service mesh is an infrastructure layer that provides features like service-to-service communication, load balancing, and security for microservices.
Think of a service mesh as a network of interconnected communication channels within a company, ensuring secure and efficient data flow between departments.
Conceptual with Istio
# Example of an Istio VirtualService configuration
apiVersion: networking.istio.io/v1beta1
kind: VirtualService
metadata:
name: example-virtualservice
spec:
hosts:
- example-service
http:
- route:
- destination:
host: example-service
port:
number: 80
In this code, you can see how Istio’s VirtualService configuration is used to define the routing of HTTP traffic within a microservices architecture. Istio is a popular service mesh that helps manage microservices traffic, security, and observability in a Kubernetes environment.
A VirtualService is one of Istio’s core components and is used to control how traffic is directed to specific services within the mesh.
The configuration starts with the apiVersion
and kind
fields, which specify that this is an Istio VirtualService
resource and the API version used to define it. The metadata
section gives the virtual service a name, example-virtualservice
, which can be used to reference it within the Istio mesh.
The spec
section defines the main functionality of the VirtualService. The hosts
field lists the services that this VirtualService applies to—in this case, it specifies a service called example-service
.
This is the destination for the traffic that matches the routing rules defined within this VirtualService.
In the http
section, we define how HTTP traffic should be routed. The route
field specifies that requests to the example-service
should be forwarded to the host example-service
on port 80. This is a basic routing rule where all incoming HTTP traffic that matches the example-service
will be directed to the service on port 80. More complex routing rules could be added here, such as load balancing between multiple instances of a service, routing based on request headers, or applying retries and timeouts.
This example is a simple yet powerful demonstration of Istio’s traffic management capabilities. Istio enables fine-grained control over how microservices communicate with each other, making it possible to implement advanced traffic routing strategies such as A/B testing, blue-green deployments, and canary releases.
- Benefits: Simplified communication management, security, and observability.
- Challenges: Additional complexity in setup and management.
It’s like using a company-wide intranet to manage internal communication, which adds layers of control but requires proper setup.
- Better Integration with CI/CD: Improved integration of service meshes with continuous integration and deployment pipelines.
- Advanced Security Features: Enhanced mechanisms for securing service-to-service communication.
Artificial Intelligence and Machine Learning Integration
Incorporating AI and machine learning into microservices to enable predictive analytics, automation, and intelligent decision-making.
It’s like adding a personal assistant to your team that can analyze data and provide recommendations or automate repetitive tasks.
Using TensorFlow.js
const tf = require('@tensorflow/tfjs');
// Define a simple model
const model = tf.sequential();
model.add(tf.layers.dense({ units: 1, inputShape: [1] }));
model.compile({ optimizer: 'sgd', loss: 'meanSquaredError' });
// Training data
const xs = tf.tensor1d([1, 2, 3, 4]);
const ys = tf.tensor1d([1, 3, 5, 7]);
// Train the model
model.fit(xs, ys, { epochs: 10 }).then(() => {
model.predict(tf.tensor1d([5])).print(); // Predict new values
});
The above example demonstrates how TensorFlow.js is used to define and train a simple machine learning model in Javascript. TensorFlow.js is a popular library that allows you to train and deploy machine learning models directly in the browser or in Node.js environments.
This example demonstrates how to create a model, train it with some data, and make predictions using that model.
The first line imports the TensorFlow.js library (const tf = require('@tensorflow/tfjs');
), making its functionality available for use in this script. TensorFlow.js provides a rich set of APIs for building, training, and evaluating machine learning models.
The code then proceeds to define a simple machine learning model using the tf.sequential()
function, which creates a linear stack of layers. This is a simple model composed of a single layer: a dense layer (tf.layers.dense
). The dense layer has 1 unit and expects an input shape of 1, meaning it will take in a single numeric input per training sample.
Once the model structure is defined, it is compiled with the model.compile()
method. This step sets up the model for training by specifying the optimizer and loss function. The optimizer: 'sgd'
indicates that stochastic gradient descent (SGD) will be used to update the model's weights during training.
The loss: 'meanSquaredError'
specifies that the model will minimize the mean squared error (MSE) during training, which is commonly used for regression tasks (where the goal is to predict continuous values).
Next, the training data is defined. The input data (xs
) is a 1-dimensional tensor with the values [1, 2, 3, 4]
, and the target output data (ys
) is another tensor with the corresponding values [1, 3, 5, 7]
. This dataset suggests a simple linear relationship: y = 2x - 1
.
The model is trained using the model.fit()
function. This method takes in the training data (xs
, ys
) and the number of epochs (iterations) to train for. In this case, the model is trained for 10 epochs. During each epoch, the model updates its internal weights to minimize the loss function (mean squared error). After training, the model is capable of making predictions.
Finally, after the model is trained, the model.predict()
function is called with new input data (tf.tensor1d([5])
). This predicts the output for an unseen input (in this case, x = 5
). The print()
method is used to display the predicted result.
Through this code, you can see how TensorFlow.js provides an easy and flexible way to create, train, and use machine learning models in JavaScript.
The model here performs a simple linear regression, but TensorFlow.js can be used to tackle much more complex tasks, including deep learning and neural networks, in both the browser and server-side environments.
- Benefits: Enhanced capabilities such as predictive analytics, automation, and personalized user experiences.
- Challenges: Complexity in integrating AI/ML models, and the need for large datasets and computational resources.
It’s like hiring a data scientist who can provide insights and automate processes but requires careful integration and resources.
- Increased Use of AutoML: Simplified processes for training and deploying machine learning models.
- More Advanced AI Models: Incorporation of more sophisticated models and techniques for various use cases.
Edge Computing
Edge computing involves processing data closer to the data source (for example, IoT devices) rather than relying solely on centralized cloud servers.
Like having a local technician who can handle immediate issues on-site rather than sending everything to a central repair facility.
- Benefits: Reduced latency, improved performance, and decreased bandwidth usage.
- Challenges: Complexity in managing distributed edge devices and ensuring data consistency.
It’s like managing multiple local warehouses to reduce shipping times, but requiring coordination and consistency.
- More Advanced Edge Devices: Development of more powerful and intelligent edge devices.
- Improved Data Management: Enhanced tools for managing and syncing data across edge and central systems.
Enhanced Security Practices
Implementation of advanced security practices such as zero-trust models, encryption, and secure APIs to protect microservices.
It’s like having a comprehensive security system with surveillance, access control, and encryption to protect your premises and data.
Using Crypto for Encryption
const crypto = require('crypto');
// Encrypt data
function encrypt(text) {
const cipher = crypto.createCipher('aes-256-cbc', 'password');
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
return encrypted;
}
// Decrypt data
function decrypt(text) {
const decipher = crypto.createDecipher('aes-256-cbc', 'password');
let decrypted = decipher.update(text, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
const text = 'Hello World';
const encryptedText = encrypt(text);
const decryptedText = decrypt(encryptedText);
console.log('Encrypted:', encryptedText);
console.log('Decrypted:', decryptedText);
This code exhibits how encryption and decryption are implemented in Node.js using the crypto
module, which provides a variety of cryptographic functionality, including hashing, signing, and encryption.
The encryption used here follows the AES-256-CBC algorithm, which is a widely used symmetric encryption algorithm. This means that the same key is used for both encryption and decryption.
The encrypt()
function demonstrates the process of encrypting a plain text message. It first creates a cipher instance using the crypto.createCipher()
method, specifying aes-256-cbc
as the encryption algorithm and 'password'
as the encryption key. The createCipher()
method returns a cipher object that is used to process the text.
The encryption process is done in two stages. First, the cipher.update()
method is used to encrypt the input text, in this case 'Hello World'
. The method takes three arguments: the input text, the encoding of the input text (here it's 'utf8'
), and the encoding of the output (here it's 'hex'
).
This means the encrypted text will be output in hexadecimal format. The second part, cipher.final('hex')
, ensures the final padding and encryption are properly applied, returning the complete encrypted text. This encrypted string is returned as the result of the encrypt()
function.
The decrypt()
function works similarly but in reverse. It starts by creating a decipher instance using crypto.createDecipher()
, again specifying 'aes-256-cbc'
as the algorithm and the same key ('password'
).
The decipher.update()
method is used to decrypt the data, converting it back from hexadecimal format to UTF-8. As with the encryption function, decipher.final('utf8')
ensures the complete decryption of the data, returning the decrypted string.
In the example, the text 'Hello World'
is first encrypted and then immediately decrypted. The output demonstrates how the original text is converted into an encrypted format and then restored back to its original form.
The use of 'password'
as a static key in this example is not secure for real-world applications, but it serves to illustrate the basic encryption and decryption process.
This example also highlights the importance of using strong, unique keys for cryptographic operations in practice, as well as ensuring that encrypted data is safely stored and transmitted.
The crypto
module, which is built into Node.js, makes it easy to implement secure encryption and decryption in any application requiring data protection.
- Benefits: Enhanced protection against data breaches and cyber-attacks.
- Challenges: Increased complexity in implementation and management.
It’s like upgrading from a basic lock to a high-security system with multiple layers of protection.
- Zero Trust Architectures: Increased adoption of zero trust models where verification is required for every request.
- Advanced Encryption Techniques: Continued development of more secure and efficient encryption methods.
Multi-Cloud and Hybrid Cloud Strategies
Using multiple cloud providers (multi-cloud) or combining on-premises infrastructure with cloud services (hybrid cloud) to improve flexibility and avoid vendor lock-in.
It’s like having accounts with multiple banks to take advantage of different services and avoid reliance on a single provider.
Conceptual with Multiple Cloud Providers
// Example of interacting with multiple cloud providers
const AWS = require('aws-sdk');
const azure = require('azure-storage');
// AWS S3 interaction
const s3 = new AWS.S3();
s3.listBuckets((err, data) => {
if (err) console.log(err, err.stack);
else console.log('S3 Buckets:', data.Buckets);
});
// Azure Blob Storage interaction
const blobService = azure.createBlobService();
blobService.listContainers((err, result) => {
if (err) console.log(err);
else console.log('Azure Containers:', result.entries);
});
This code describes how you can interact with two distinct cloud providers—AWS and Azure—specifically their storage services. The code demonstrates how to use AWS S3 and Azure Blob Storage APIs to list buckets and containers, respectively.
The first part of the code shows how to interact with AWS S3. It imports the aws-sdk
package, which is a Node.js SDK that allows applications to interact with AWS services.
A new instance of the S3
service is created using new AWS.S3()
. The listBuckets()
method is then called on the S3
instance to retrieve a list of all buckets within the configured AWS account.
This method is asynchronous, so it takes a callback function as an argument. If the operation is successful, the callback logs the list of buckets to the console. If there's an error, the error message is printed instead.
This demonstrates a basic interaction with AWS's S3 service, where you can programmatically access and manage your storage containers (called "buckets").
Next, the code switches to Azure Blob Storage. It uses the azure-storage
package, which is the official SDK for interacting with Azure's storage services. The createBlobService()
method is used to create a blob service client that interacts with Azure Blob Storage.
The listContainers()
method is called on the blob service client to list all the containers in the account. As with AWS, this method is asynchronous, and the result is provided via a callback. If successful, the list of containers (stored in the entries
property) is logged to the console.
This code shows how developers can integrate with multiple cloud platforms to manage cloud storage resources, using the APIs provided by each service. The primary takeaway is that both AWS and Azure provide SDKs for interacting with their services, making it easy to automate and manage cloud resources programmatically.
These APIs allow you to perform basic tasks such as listing storage containers, which is a common requirement when working with cloud storage solutions. By using these SDKs, applications can remain cloud-agnostic while still leveraging the full power of each platform’s storage offerings.
- Benefits: Greater flexibility, reduced risk of vendor lock-in, and optimization of services across providers.
- Challenges: Increased complexity in managing and integrating services across different environments.
It’s like using different suppliers for various needs to get the best deals but requiring careful coordination and management.
- Improved Integration Tools: Development of better tools and platforms for managing multi-cloud and hybrid cloud environments.
- Advanced Orchestration: Enhanced orchestration and management capabilities across diverse cloud environments.