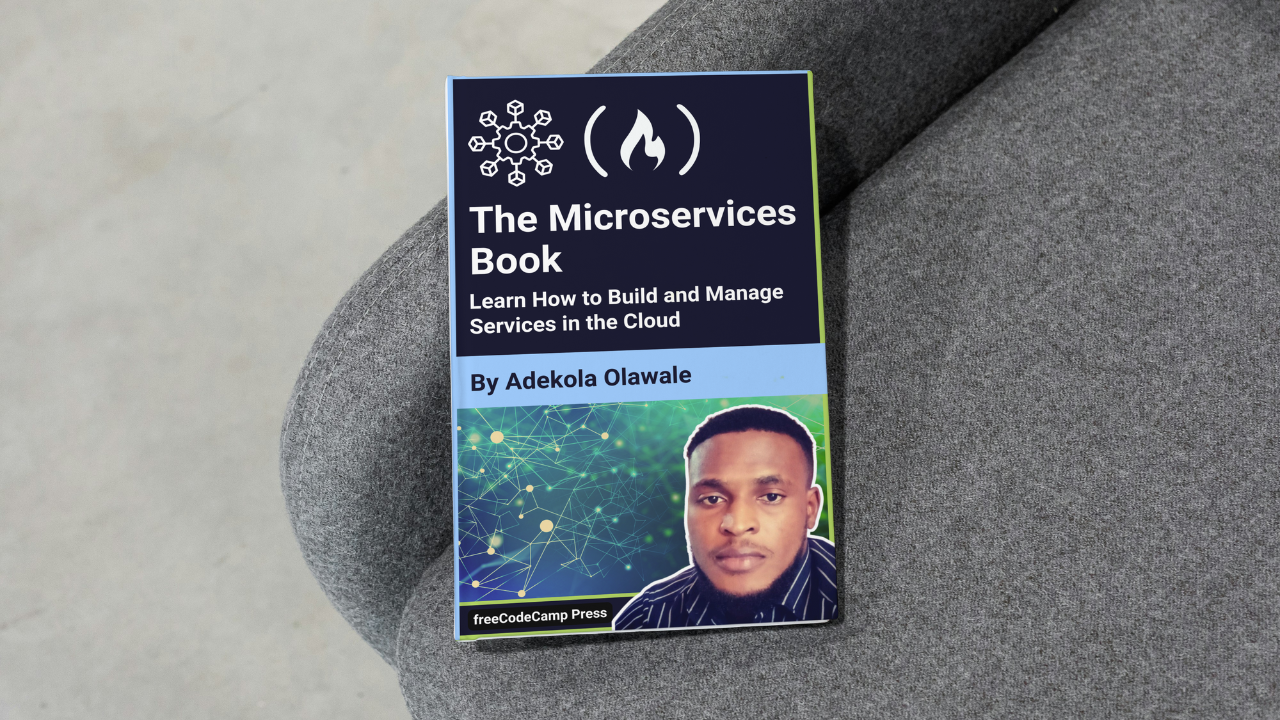
How to Deploy Microservices
How to Deploy Microservices ๊ด๋ จ
Deploying microservices efficiently is a key part of building scalable and resilient applications. As microservices are typically small, independent services, they must be deployed in a way that allows them to function together seamlessly within a larger ecosystem.
Unlike traditional monolithic applications, microservices require a different approach to deployment, focusing on automation, scalability, and continuous delivery. Deployment also involves dealing with challenges such as service discovery, load balancing, and ensuring fault tolerance.
In this section, Iโll guide you through the various strategies and tools for deploying microservices. From containerization with Docker to orchestrating services with Kubernetes, weโll explore how these technologies simplify the deployment process.
We will also cover essential topics such as continuous integration/continuous deployment (CI/CD) pipelines, automated scaling, and monitoring to ensure that your microservices architecture remains robust and adaptable in production environments.
By the end of this section, you will have a clear understanding of how to deploy microservices efficiently and how to maintain them as your application grows.
Containerization with Docker
Packaging microservices into Docker containers helps you consistently deploy across different environments.
Itโs like using standardized shipping containers to transport goods efficiently and predictably.
# Dockerfile for a Node.js app
# Use Node.js image
FROM node:14
# Set working directory
WORKDIR /usr/src/app
# Copy package.json and install dependencies
COPY package*.json ./
RUN npm install
# Copy application code
COPY . .
# Expose port
EXPOSE 3000
# Run the application
CMD [ "node", "app.js" ]
Here, the code illustrates how you can use Docker to create a containerized environment for a Node.js application, ensuring that it can be deployed consistently across different environments.
Containerization with Docker works by encapsulating all the necessary application components, like code, runtime, libraries, and dependencies, into a standardized container image.
This approach provides predictable, repeatable deployments, similar to how standardized shipping containers are used to transport goods reliably across various transportation systems.
Starting with FROM node:14
, the Dockerfile specifies a base image, in this case, an official Node.js image with version 14. This base image provides a pre-configured environment with Node.js installed, reducing the setup time and complexity required to run the app.
By using a standardized base, this Dockerfile also ensures compatibility and eliminates potential inconsistencies that could occur with different Node.js versions.
The WORKDIR /usr/src/app
command sets the working directory inside the container to /usr/src/app
, which organizes the applicationโs code files and simplifies file path references later in the Dockerfile.
The COPY package*.json ./
line then copies the package.json
files into this working directory, and RUN npm install
installs the necessary Node.js dependencies. This process isolates the dependency installation to ensure that all required libraries are present, matching the exact versions defined in package.json
.
Next, COPY . .
copies the rest of the application files from the host system into the containerโs working directory.
The EXPOSE 3000
command designates port 3000 as the applicationโs external communication port, allowing traffic to be directed to this port when the container is run. Finally, CMD ["node", "app.js"]
defines the containerโs entry point, instructing Docker to execute node app.js
to start the application when the container is launched.
This Dockerfile showcases the fundamental steps in building a Docker image for a Node.js app, enabling consistent and reproducible deployments. By following these steps, developers ensure that the application can be easily transferred between development, testing, and production environments without compatibility issues.
This predictable deployment approach streamlines operations, making it ideal for scaling and managing microservices in a production ecosystem.