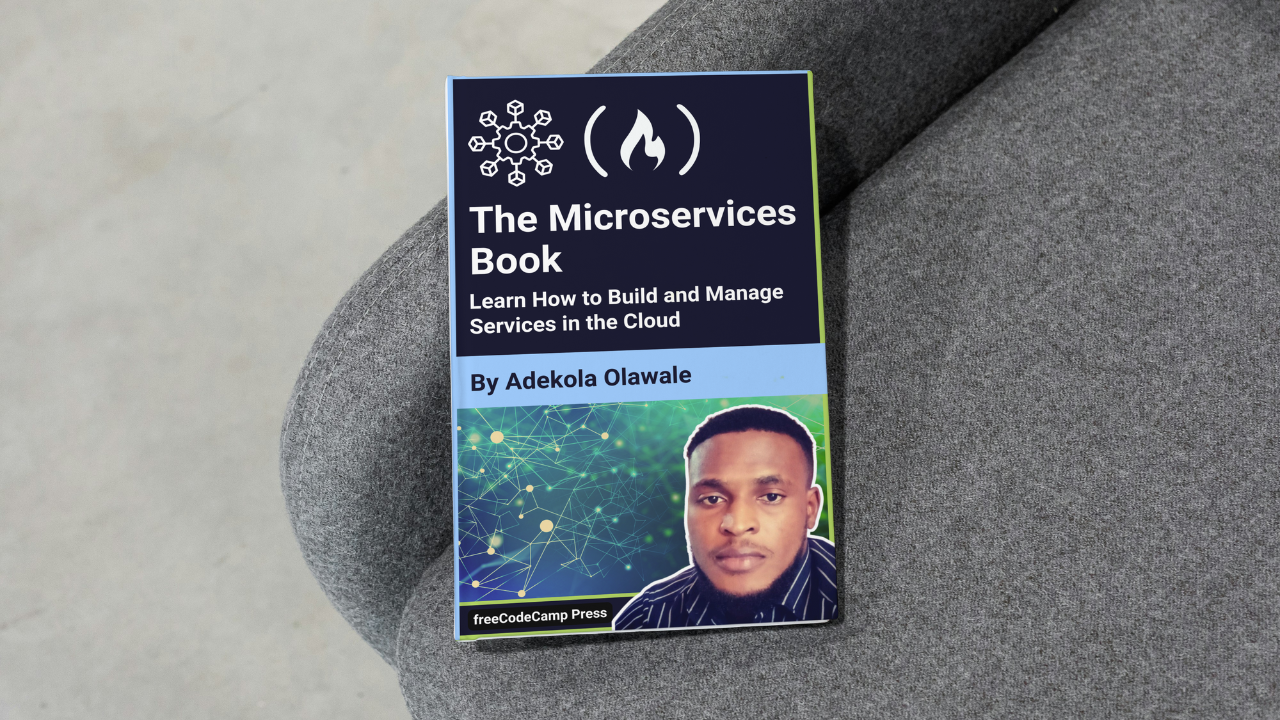
How to Test Microservices
How to Test Microservices 관련
Testing is an essential part of ensuring the reliability, scalability, and performance of microservices. Given that microservices are composed of multiple independent services that communicate over the network, rigorous testing becomes even more critical.
With each service potentially evolving independently, it’s crucial to identify and address issues early to prevent cascading failures and disruptions in the overall system. Without comprehensive testing, microservices can become prone to hidden bugs, integration issues, and performance bottlenecks.
In this section, we’ll explore the different types of testing that are important for microservices. Each type serves a specific purpose, from validating individual components to ensuring that the entire system works together as expected.
You'll learn how to apply unit testing, integration testing, contract testing, and end-to-end testing to create a robust and reliable microservice-based architecture.
By the end of this section, you'll understand how to approach testing in a microservices environment, enabling you to deliver high-quality applications.
Unit Testing
Testing individual components of a microservice is important to ensure that they work correctly in isolation.
This is like testing each part of a machine separately to ensure each part functions properly before assembling the entire machine.
// Using Mocha and Chai
const { expect } = require('chai');
const UserService = require('./userService'); // Assume UserService is in another file
describe('UserService', () => {
let userService;
beforeEach(() => {
userService = new UserService();
});
it('should create a user', () => {
const user = { id: 1, name: 'John Doe' };
userService.createUser(user);
expect(userService.getUser(1)).to.deep.equal(user);
});
});
This code demonstrates how you can use Mocha and Chai to perform unit testing on the UserService
class. The purpose of this test is to verify that the UserService
class's createUser
and getUser
methods work as expected, ensuring that individual components of this microservice are reliable when tested in isolation.
This is essential for microservices, where each component must be robust to ensure that the system as a whole functions smoothly.
Here, the test suite begins with describe('UserService', ...)
, which serves as a container for grouping multiple related test cases about UserService
. Inside the suite, a new instance of UserService
is created before each test by using the beforeEach()
function, which resets the state of the userService
instance, making each test independent and repeatable.
The actual test case, it('should create a user', ...)
, simulates adding a user to the service. It defines a user object, { id: 1, name: 'John Doe' }
, which it then passes to createUser
.
The expect
assertion from Chai is used to compare the result of userService.getUser(1)
to the expected user
object.
By using deep.equal
, the test confirms that the user retrieved by getUser
has the same properties as the user added by createUser
, checking both the ID and name fields.
This test validates that each part of UserService
works as intended, fulfilling the principle of unit testing by ensuring components function correctly in isolation.
This approach is analogous to testing individual parts of a machine separately to ensure reliability before integrating them into the larger system, helping catch issues at the component level early in the development process.
Integration Testing
Integration testing involves testing the interactions between microservices to ensure that they work together correctly.
It’s like testing different departments in a company to ensure their workflows align and function seamlessly together.
const request = require('supertest');
const app = require('./app'); // Assume app is your Express application
describe('Integration Tests', () => {
it('should create and retrieve a user', async () => {
const user = { id: 1, name: 'Jane Doe' };
// Test creating a user
await request(app)
.post('/users')
.send(user)
.expect(201);
// Test retrieving the user
const response = await request(app)
.get('/users/1')
.expect(200);
expect(response.body).to.deep.equal(user);
});
});
In this code, you can see how integration testing is performed using the Supertest library to verify interactions within the Express application. Integration testing is crucial for microservices as it checks that different components work correctly together, just as different departments in a company need to collaborate seamlessly.
The code defines a test suite describe('Integration Tests', ...)
, where Supertest is used to make HTTP requests to the Express app and assert the responses. First, it tests creating a user by sending a POST
request to /users
with user data, { id: 1, name: 'Jane Doe' }
, which is expected to return a status code 201
, indicating successful creation.
The test then proceeds to check if this user can be retrieved by making a GET
request to /users/1
. This call is expected to return a 200
status, confirming that the user retrieval is functioning as expected.
The expect
assertion is used here to ensure the response data (response.body
) matches the created user data, { id: 1, name: 'Jane Doe' }
. This comparison validates that the app correctly processes and returns data across different endpoints, verifying that the service’s internal workflows are cohesive.
This approach of combining Supertest and assertions provides a reliable way to validate that the app's interconnected parts work as intended, allowing for early detection of issues that could disrupt service integrations in real-world deployments.
End-to-End Testing
End-to-End testing makes sure that the entire application works from start to finish and checks that all components work together as expected.
It’s like running a full simulation of a business process to ensure everything from start to finish operates correctly.
// Using Cypress
describe('End-to-End Test', () => {
it('should create a user and verify its details', () => {
cy.request('POST', '/users', { id: 1, name: 'Jack Doe' })
.then(response => {
expect(response.status).to.eq(201);
});
cy.request('/users/1')
.then(response => {
expect(response.status).to.eq(200);
expect(response.body).to.have.property('name', 'Jack Doe');
});
});
});
This code illustrates how you can use Cypress to conduct an end-to-end test of a microservice application.
The test suite, named describe('End-to-End Test', ...)
, is designed to create a user and verify its details. The cy.request
method is used to simulate HTTP requests, interacting with the application’s endpoints as a real client would.
First, it sends a POST
request to the /users
endpoint, adding a user with { id: 1, name: 'Jack Doe' }
. After this request, an assertion checks that the response status is 201
, indicating the successful creation of the user resource.
The test then moves to the second part, where it retrieves the user with cy.request('/users/1')
. The test verifies that the status code is 200
, meaning the user was found successfully. Also, expect(response.body).to.have.property('name', 'Jack Doe')
confirms that the user’s name property matches the expected value, 'Jack Doe'
.
This test validates the entire flow of creating and retrieving a user in the system, ensuring that the application’s different components, such as database interactions and HTTP request handling, function cohesively.
Cypress is particularly effective for E2E testing because it runs these requests in a controlled environment, allowing developers to test real-world scenarios with reliable assertions. This type of testing can catch integration issues that may not appear in unit or integration tests, providing greater confidence in the system's overall stability.