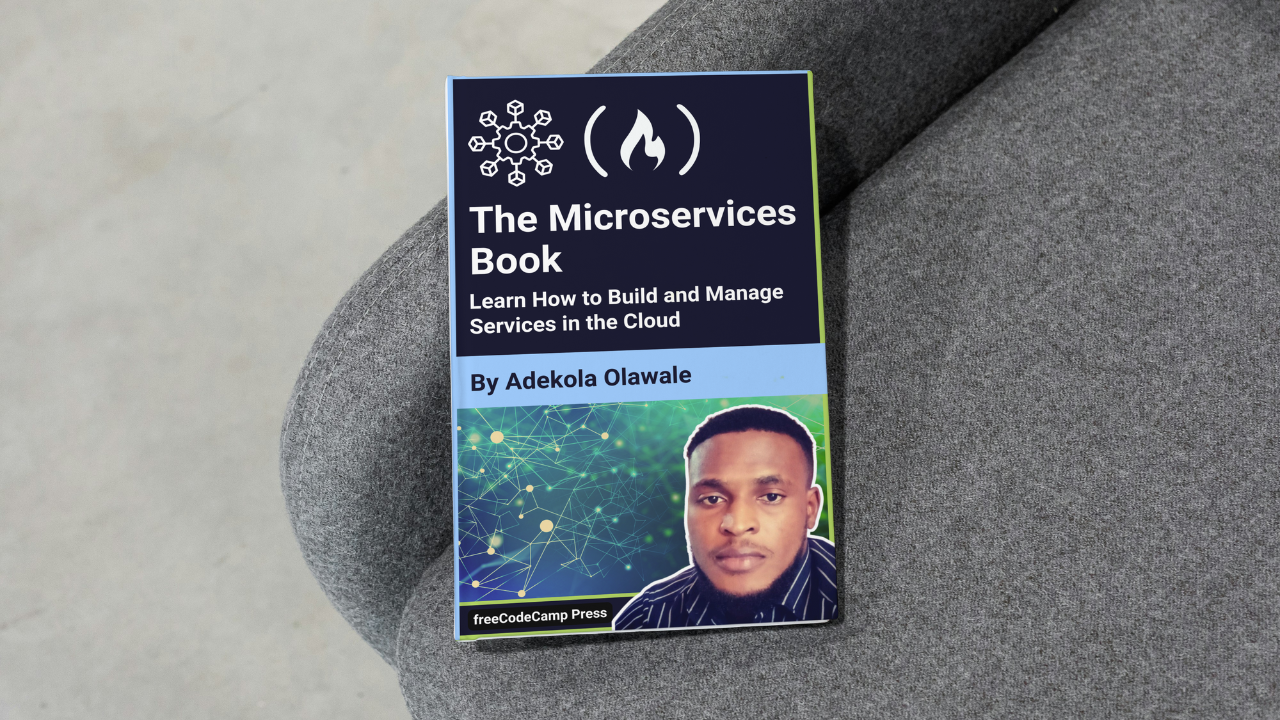
Monitoring and Logging
Monitoring and Logging κ΄λ ¨
Effective monitoring and logging are fundamental to maintaining the health and performance of a microservices-based application. As microservices often operate in distributed environments, it becomes challenging to track, diagnose, and troubleshoot issues. Without proper visibility into the systemβs behavior, you risk operational inefficiencies, performance bottlenecks, and increased downtime.
In this section, we will focus on how to implement robust monitoring and logging practices that ensure you can effectively track and manage the behavior of microservices in real-time.
We'll explore the tools and frameworks available for monitoring system health, gathering performance metrics, and collecting logs from different microservices in your application.
We'll also discuss how these practices can support proactive issue resolution by allowing for timely alerts and more insightful data for debugging.
Then weβll dive into the importance of centralized logging systems like ELK Stack (Elasticsearch, Logstash, and Kibana), and how monitoring solutions such as Prometheus and Grafana provide metrics and visualizations to observe your services' health.
Finally, weβll cover tracing techniques that can help pinpoint the flow of requests across microservices, ensuring quick resolution of performance or failure issues.
By the end of this section, you'll understand how to implement a comprehensive monitoring and logging strategy that ensures your microservices architecture operates smoothly and reliably.
Centralized Logging Solutions (ELK Stack, Fluentd)
Microservices generate logs across many instances. Centralized logging solutions collect and store logs in a single location, simplifying analysis.
- ELK Stack (Elasticsearch, Logstash, Kibana): Common for centralized logging, enabling full-text search and visualizations.
Monitoring and Observability Tools (Prometheus, Grafana, Datadog)
Monitoring tools track the performance and health of microservices. Prometheus collects metrics, and Grafana visualizes them in dashboards.
Prometheus (Monitoring Node.js Microservice)
const client = require('prom-client');
// Create a counter metric
const requestCounter = new client.Counter({
name: 'node_requests_total',
help: 'Total number of requests'
});
// Increment counter on each request
app.use((req, res, next) => {
requestCounter.inc();
next();
});
The following code shows the process of how Prometheus metrics are integrated into a Node.js application using the prom-client
library to monitor API requests.
Prometheus is a popular tool for monitoring and alerting in microservices environments, often used to track and visualize system health metrics like request counts, response times, and error rates.
Here, the code is focused on implementing a simple counter metric to monitor the total number of requests the application receives.
First, the prom-client
module is imported to set up Prometheus-compatible metrics in the application. The Counter
class from prom-client
is used to define a new counter metric, named node_requests_total
, with a description (via the help
property) of "Total number of requests."
Counters in Prometheus are designed for tracking cumulative values, like the count of requests or the number of errors, and are ideal for metrics that always increase, such as a request count.
The middleware function then increments this counter on every incoming request by calling requestCounter.inc()
. This middleware is added to the Express app
instance using app.use()
, which means it will execute for every incoming request, incrementing the requestCounter
metric.
Each time a new request is processed, Prometheus records this increment, allowing the total count of requests to be monitored over time.
This setup allows Prometheus to pull these metrics at regular intervals from the applicationβs /metrics
endpoint (if configured).
By tracking the node_requests_total
counter, you can gain insights into traffic patterns and detect sudden increases or decreases in request volume, which can be crucial for monitoring system performance and ensuring service reliability.
This basic example demonstrates how to set up and use Prometheus metrics to gain visibility into microservice activity
Distributed Tracing (Jaeger, Zipkin)
In microservices, tracking a request's journey across services is crucial. Distributed tracing tools like Jaeger and Zipkin provide visibility into how requests propagate across services.
Distributed tracing is like tracking a packageβs journey through multiple shipping hubs, providing insights into where delays occur.